An introduction to GraphQL with Python
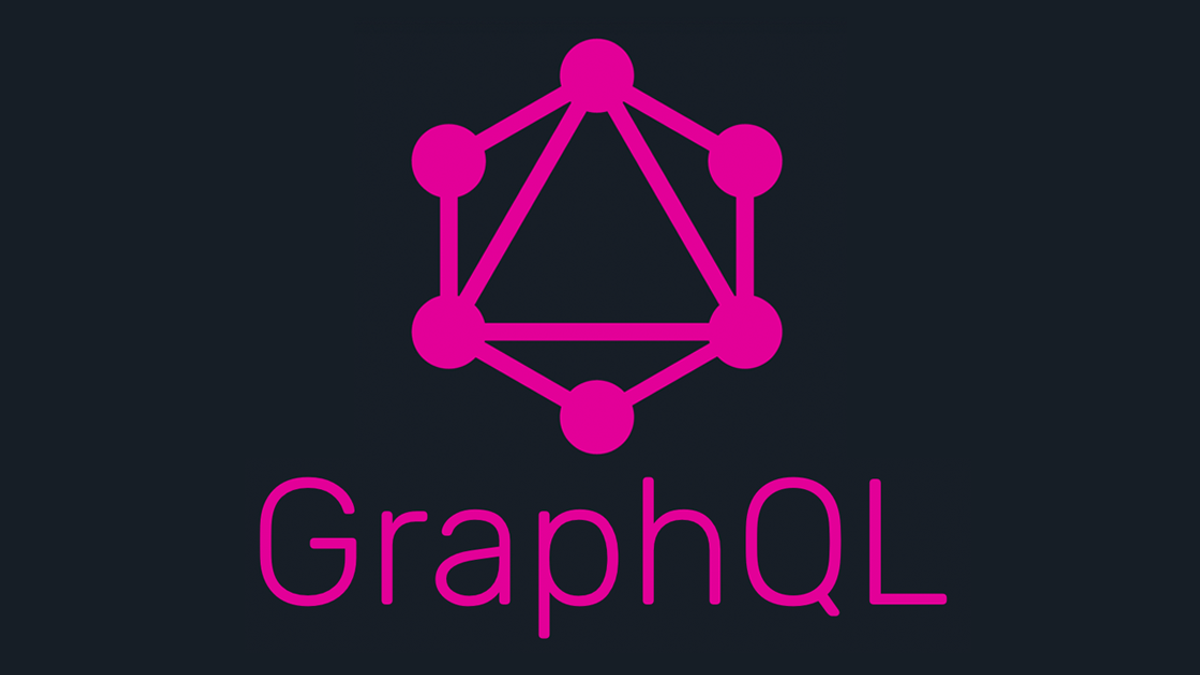
GraphQL is a relatively new API interface that is rapidly gaining traction. The major benefit of a GraphQL is that a single query can get information about a much larger number of elements than a standard REST API. This means that queries can be much more efficient. For a rest API we need to hit different endpoints to get data about different topics. For a GraphQL query we can just include all the things that we want to know in one query. This makes the code we have to write a lot smaller and a lot easier to understand. In this section we are going to use an open GraphQL endpoint to demonstrate how to get data from one for the start of a data science project.
For this example we are going to use an open GraphQL API for information on the TV show "Rick and Morty." You can find all the info about the API at https://rickandmortyapi.com/. Convieniently, this site actually has both a GraphQL and a REST API. This will let us demonstrate some of the differences between the two.
For this example we are going to start out by importing the request
library again, since we can actually use the requests
library for both the GraphQL and REST calls. Then we are going to create two variable, one is the BASE_URL
where we put in the GraphQL endpoint and the other is the actual query
. Since all GraphQL APIs only have a single endpoint, we don't need all the different endpoints for different pieces of information. For our query we are going to start of prety simple and then expand from there. We are going to keep all our code here, but if you are going to actually use a GraphQL API in a project there is usually a nice playground type area to try out your queries before you put them in your code. For the Rick and Morty API you can find the playground here: https://rickandmortyapi.com/graphql.
First what we want to do is try to just get the titles of all the episodes, so we will use the below query.
(Note that we are using POST for the GraphQL query, as apposed to GET that we use for REST API calls)
1import requests
2import json
3
4BASE_URL = 'https://rickandmortyapi.com/graphql'
5
6query = """ {
7 episodes
8}
9"""
10
11response = requests.post(BASE_URL, json={'query': query})
12json_object = response.json()
13json_formatted = json.dumps(json_object, indent=4)
14print(json_formatted)
1{
2 "errors": [
3 {
4 "message": "Field \"episodes\" of type \"Episodes\" must have a selection of subfields. Did you mean \"episodes { ... }\"?",
5 "locations": [
6 {
7 "line": 2,
8 "column": 3
9 }
10 ],
11 "extensions": {
12 "code": "GRAPHQL_VALIDATION_FAILED"
13 }
14 }
15 ]
16}
It looks like we ran into a problem though. According to the error above we need to have some selection of subfields in the query. If we go into the playground and start to interact with the query we structure it to get all the episode names by including the results
as a property of episodes
and then finally by asing for the name
of each result. This will give us the following.
1BASE_URL = 'https://rickandmortyapi.com/graphql'
2
3query = """ {
4 episodes {
5 results {
6 name
7 }
8 }
9}
10"""
11
12response = requests.post(BASE_URL, json={'query': query})
13json_object = response.json()
14json_formatted = json.dumps(json_object, indent=4)
15print(json_formatted)
1{
2 "data": {
3 "episodes": {
4 "results": [
5 {
6 "name": "Pilot"
7 },
8 {
9 "name": "Lawnmower Dog"
10 },
11 {
12 "name": "Anatomy Park"
13 },
14 {
15 "name": "M. Night Shaym-Aliens!"
16 },
17 {
18 "name": "Meeseeks and Destroy"
19 },
20 {
21 "name": "Rick Potion #9"
22 },
23 {
24 "name": "Raising Gazorpazorp"
25 },
26 {
27 "name": "Rixty Minutes"
28 },
29 {
30 "name": "Something Ricked This Way Comes"
31 },
32 {
33 "name": "Close Rick-counters of the Rick Kind"
34 },
35 {
36 "name": "Ricksy Business"
37 },
38 {
39 "name": "A Rickle in Time"
40 },
41 {
42 "name": "Mortynight Run"
43 },
44 {
45 "name": "Auto Erotic Assimilation"
46 },
47 {
48 "name": "Total Rickall"
49 },
50 {
51 "name": "Get Schwifty"
52 },
53 {
54 "name": "The Ricks Must Be Crazy"
55 },
56 {
57 "name": "Big Trouble in Little Sanchez"
58 },
59 {
60 "name": "Interdimensional Cable 2: Tempting Fate"
61 },
62 {
63 "name": "Look Who's Purging Now"
64 }
65 ]
66 }
67 }
68}
69
So now that we see we have all the data in the response, we just need to loop through the JSON we just got to print out all the episodes.
1for episode in json_object['data']['episodes']['results']:
2 print(episode['name'])
1Pilot
2Lawnmower Dog
3Anatomy Park
4M. Night Shaym-Aliens!
5Meeseeks and Destroy
6Rick Potion #9
7Raising Gazorpazorp
8Rixty Minutes
9Something Ricked This Way Comes
10Close Rick-counters of the Rick Kind
11Ricksy Business
12A Rickle in Time
13Mortynight Run
14Auto Erotic Assimilation
15Total Rickall
16Get Schwifty
17The Ricks Must Be Crazy
18Big Trouble in Little Sanchez
19Interdimensional Cable 2: Tempting Fate
20Look Who's Purging Now
If we look at the structure of the above we can see that it is actually giving us all our data in the exact same format we sent the request over in. Meaning the highest level (after data
) is episodes
, then the results
which has an array of obejects with name
s. This makes making queries and getting the data out of them very straight forward.
Now lets quickly compare this to a similar query using the REST API. To do this we need the REST API BASE_URL
and then the EPISODE_ENDPOINT
.
1BASE_URL = 'https://rickandmortyapi.com/api'
2
3CHARACTER_ENDPOINT = '/character'
4LOCATION_ENDPOINT = '/location'
5EPISODE_ENDPOINT = '/episode'
6
7
8response = requests.get(BASE_URL + EPISODE_ENDPOINT)
9json_object = response.json()
10json_formatted = json.dumps(json_object, indent=4)
11print(json_formatted)
1{
2 "info": {
3 "count": 41,
4 "pages": 3,
5 "next": "https://rickandmortyapi.com/api/episode?page=2",
6 "prev": null
7 },
8 "results": [
9 {
10 "id": 1,
11 "name": "Pilot",
12 "air_date": "December 2, 2013",
13 "episode": "S01E01",
14 "characters": [
15 "https://rickandmortyapi.com/api/character/1",
16 "https://rickandmortyapi.com/api/character/2",
17 "https://rickandmortyapi.com/api/character/35",
18 "https://rickandmortyapi.com/api/character/38",
19 "https://rickandmortyapi.com/api/character/62",
20 "https://rickandmortyapi.com/api/character/92",
21 "https://rickandmortyapi.com/api/character/127",
22 "https://rickandmortyapi.com/api/character/144",
23 "https://rickandmortyapi.com/api/character/158",
24 "https://rickandmortyapi.com/api/character/175",
25 "https://rickandmortyapi.com/api/character/179",
26 "https://rickandmortyapi.com/api/character/181",
27 "https://rickandmortyapi.com/api/character/239",
28 "https://rickandmortyapi.com/api/character/249",
29 "https://rickandmortyapi.com/api/character/271",
30 "https://rickandmortyapi.com/api/character/338",
31 "https://rickandmortyapi.com/api/character/394",
32 "https://rickandmortyapi.com/api/character/395",
33 "https://rickandmortyapi.com/api/character/435"
34 ],
35 "url": "https://rickandmortyapi.com/api/episode/1",
36 "created": "2017-11-10T12:56:33.798Z"
37 },
38 {
39 "id": 2,
40 "name": "Lawnmower Dog",
41 "air_date": "December 9, 2013",
42 "episode": "S01E02",
43 "characters": [
44 "https://rickandmortyapi.com/api/character/1",
45 "https://rickandmortyapi.com/api/character/2",
46 "https://rickandmortyapi.com/api/character/38",
47 "https://rickandmortyapi.com/api/character/46",
48 "https://rickandmortyapi.com/api/character/63",
49 "https://rickandmortyapi.com/api/character/80",
50 "https://rickandmortyapi.com/api/character/175",
51 "https://rickandmortyapi.com/api/character/221",
52 "https://rickandmortyapi.com/api/character/239",
53 "https://rickandmortyapi.com/api/character/246",
54 "https://rickandmortyapi.com/api/character/304",
55 "https://rickandmortyapi.com/api/character/305",
56 "https://rickandmortyapi.com/api/character/306",
57 "https://rickandmortyapi.com/api/character/329",
58 "https://rickandmortyapi.com/api/character/338",
59 "https://rickandmortyapi.com/api/character/396",
60 "https://rickandmortyapi.com/api/character/397",
61 "https://rickandmortyapi.com/api/character/398",
62 "https://rickandmortyapi.com/api/character/405"
63 ],
64 "url": "https://rickandmortyapi.com/api/episode/2",
65 "created": "2017-11-10T12:56:33.916Z"
66 },
67 {
68 "id": 3,
69 "name": "Anatomy Park",
70 "air_date": "December 16, 2013",
71 "episode": "S01E03",
72 "characters": [
73 "https://rickandmortyapi.com/api/character/1",
74 "https://rickandmortyapi.com/api/character/2",
75 "https://rickandmortyapi.com/api/character/12",
76 "https://rickandmortyapi.com/api/character/17",
77 "https://rickandmortyapi.com/api/character/38",
78 "https://rickandmortyapi.com/api/character/45",
79 "https://rickandmortyapi.com/api/character/96",
80 "https://rickandmortyapi.com/api/character/97",
81 "https://rickandmortyapi.com/api/character/98",
82 "https://rickandmortyapi.com/api/character/99",
83 "https://rickandmortyapi.com/api/character/100",
84 "https://rickandmortyapi.com/api/character/101",
85 "https://rickandmortyapi.com/api/character/108",
86 "https://rickandmortyapi.com/api/character/112",
87 "https://rickandmortyapi.com/api/character/114",
88 "https://rickandmortyapi.com/api/character/169",
89 "https://rickandmortyapi.com/api/character/175",
90 "https://rickandmortyapi.com/api/character/186",
91 "https://rickandmortyapi.com/api/character/201",
92 "https://rickandmortyapi.com/api/character/268",
93 "https://rickandmortyapi.com/api/character/300",
94 "https://rickandmortyapi.com/api/character/302",
95 "https://rickandmortyapi.com/api/character/338",
96 "https://rickandmortyapi.com/api/character/356"
97 ],
98 "url": "https://rickandmortyapi.com/api/episode/3",
99 "created": "2017-11-10T12:56:34.022Z"
100 },
101 {
102 "id": 4,
103 "name": "M. Night Shaym-Aliens!",
104 "air_date": "January 13, 2014",
105 "episode": "S01E04",
106 "characters": [
107 "https://rickandmortyapi.com/api/character/1",
108 "https://rickandmortyapi.com/api/character/2",
109 "https://rickandmortyapi.com/api/character/38",
110 "https://rickandmortyapi.com/api/character/87",
111 "https://rickandmortyapi.com/api/character/175",
112 "https://rickandmortyapi.com/api/character/179",
113 "https://rickandmortyapi.com/api/character/181",
114 "https://rickandmortyapi.com/api/character/191",
115 "https://rickandmortyapi.com/api/character/239",
116 "https://rickandmortyapi.com/api/character/241",
117 "https://rickandmortyapi.com/api/character/270",
118 "https://rickandmortyapi.com/api/character/337",
119 "https://rickandmortyapi.com/api/character/338"
120 ],
121 "url": "https://rickandmortyapi.com/api/episode/4",
122 "created": "2017-11-10T12:56:34.129Z"
123 },
124 {
125 "id": 5,
126 "name": "Meeseeks and Destroy",
127 "air_date": "January 20, 2014",
128 "episode": "S01E05",
129 "characters": [
130 "https://rickandmortyapi.com/api/character/1",
131 "https://rickandmortyapi.com/api/character/2",
132 "https://rickandmortyapi.com/api/character/38",
133 "https://rickandmortyapi.com/api/character/41",
134 "https://rickandmortyapi.com/api/character/89",
135 "https://rickandmortyapi.com/api/character/116",
136 "https://rickandmortyapi.com/api/character/117",
137 "https://rickandmortyapi.com/api/character/120",
138 "https://rickandmortyapi.com/api/character/175",
139 "https://rickandmortyapi.com/api/character/193",
140 "https://rickandmortyapi.com/api/character/238",
141 "https://rickandmortyapi.com/api/character/242",
142 "https://rickandmortyapi.com/api/character/271",
143 "https://rickandmortyapi.com/api/character/303",
144 "https://rickandmortyapi.com/api/character/326",
145 "https://rickandmortyapi.com/api/character/333",
146 "https://rickandmortyapi.com/api/character/338",
147 "https://rickandmortyapi.com/api/character/343",
148 "https://rickandmortyapi.com/api/character/399",
149 "https://rickandmortyapi.com/api/character/400"
150 ],
151 "url": "https://rickandmortyapi.com/api/episode/5",
152 "created": "2017-11-10T12:56:34.236Z"
153 },
154 {
155 "id": 6,
156 "name": "Rick Potion #9",
157 "air_date": "January 27, 2014",
158 "episode": "S01E06",
159 "characters": [
160 "https://rickandmortyapi.com/api/character/1",
161 "https://rickandmortyapi.com/api/character/2",
162 "https://rickandmortyapi.com/api/character/3",
163 "https://rickandmortyapi.com/api/character/4",
164 "https://rickandmortyapi.com/api/character/5",
165 "https://rickandmortyapi.com/api/character/38",
166 "https://rickandmortyapi.com/api/character/58",
167 "https://rickandmortyapi.com/api/character/82",
168 "https://rickandmortyapi.com/api/character/83",
169 "https://rickandmortyapi.com/api/character/92",
170 "https://rickandmortyapi.com/api/character/155",
171 "https://rickandmortyapi.com/api/character/175",
172 "https://rickandmortyapi.com/api/character/179",
173 "https://rickandmortyapi.com/api/character/181",
174 "https://rickandmortyapi.com/api/character/216",
175 "https://rickandmortyapi.com/api/character/234",
176 "https://rickandmortyapi.com/api/character/239",
177 "https://rickandmortyapi.com/api/character/249",
178 "https://rickandmortyapi.com/api/character/251",
179 "https://rickandmortyapi.com/api/character/271",
180 "https://rickandmortyapi.com/api/character/293",
181 "https://rickandmortyapi.com/api/character/338",
182 "https://rickandmortyapi.com/api/character/343",
183 "https://rickandmortyapi.com/api/character/394"
184 ],
185 "url": "https://rickandmortyapi.com/api/episode/6",
186 "created": "2017-11-10T12:56:34.339Z"
187 },
188 {
189 "id": 7,
190 "name": "Raising Gazorpazorp",
191 "air_date": "March 10, 2014",
192 "episode": "S01E07",
193 "characters": [
194 "https://rickandmortyapi.com/api/character/1",
195 "https://rickandmortyapi.com/api/character/2",
196 "https://rickandmortyapi.com/api/character/3",
197 "https://rickandmortyapi.com/api/character/4",
198 "https://rickandmortyapi.com/api/character/5",
199 "https://rickandmortyapi.com/api/character/59",
200 "https://rickandmortyapi.com/api/character/151",
201 "https://rickandmortyapi.com/api/character/168",
202 "https://rickandmortyapi.com/api/character/211",
203 "https://rickandmortyapi.com/api/character/230",
204 "https://rickandmortyapi.com/api/character/258",
205 "https://rickandmortyapi.com/api/character/329",
206 "https://rickandmortyapi.com/api/character/376",
207 "https://rickandmortyapi.com/api/character/401"
208 ],
209 "url": "https://rickandmortyapi.com/api/episode/7",
210 "created": "2017-11-10T12:56:34.441Z"
211 },
212 {
213 "id": 8,
214 "name": "Rixty Minutes",
215 "air_date": "March 17, 2014",
216 "episode": "S01E08",
217 "characters": [
218 "https://rickandmortyapi.com/api/character/1",
219 "https://rickandmortyapi.com/api/character/2",
220 "https://rickandmortyapi.com/api/character/3",
221 "https://rickandmortyapi.com/api/character/4",
222 "https://rickandmortyapi.com/api/character/5",
223 "https://rickandmortyapi.com/api/character/20",
224 "https://rickandmortyapi.com/api/character/28",
225 "https://rickandmortyapi.com/api/character/29",
226 "https://rickandmortyapi.com/api/character/34",
227 "https://rickandmortyapi.com/api/character/37",
228 "https://rickandmortyapi.com/api/character/54",
229 "https://rickandmortyapi.com/api/character/88",
230 "https://rickandmortyapi.com/api/character/91",
231 "https://rickandmortyapi.com/api/character/129",
232 "https://rickandmortyapi.com/api/character/134",
233 "https://rickandmortyapi.com/api/character/136",
234 "https://rickandmortyapi.com/api/character/145",
235 "https://rickandmortyapi.com/api/character/153",
236 "https://rickandmortyapi.com/api/character/157",
237 "https://rickandmortyapi.com/api/character/176",
238 "https://rickandmortyapi.com/api/character/183",
239 "https://rickandmortyapi.com/api/character/184",
240 "https://rickandmortyapi.com/api/character/195",
241 "https://rickandmortyapi.com/api/character/207",
242 "https://rickandmortyapi.com/api/character/214",
243 "https://rickandmortyapi.com/api/character/222",
244 "https://rickandmortyapi.com/api/character/250",
245 "https://rickandmortyapi.com/api/character/266",
246 "https://rickandmortyapi.com/api/character/277",
247 "https://rickandmortyapi.com/api/character/279",
248 "https://rickandmortyapi.com/api/character/314",
249 "https://rickandmortyapi.com/api/character/315",
250 "https://rickandmortyapi.com/api/character/316",
251 "https://rickandmortyapi.com/api/character/317",
252 "https://rickandmortyapi.com/api/character/318",
253 "https://rickandmortyapi.com/api/character/351",
254 "https://rickandmortyapi.com/api/character/358",
255 "https://rickandmortyapi.com/api/character/367",
256 "https://rickandmortyapi.com/api/character/370",
257 "https://rickandmortyapi.com/api/character/373",
258 "https://rickandmortyapi.com/api/character/402",
259 "https://rickandmortyapi.com/api/character/403",
260 "https://rickandmortyapi.com/api/character/404",
261 "https://rickandmortyapi.com/api/character/405",
262 "https://rickandmortyapi.com/api/character/406",
263 "https://rickandmortyapi.com/api/character/407",
264 "https://rickandmortyapi.com/api/character/408",
265 "https://rickandmortyapi.com/api/character/409",
266 "https://rickandmortyapi.com/api/character/410",
267 "https://rickandmortyapi.com/api/character/411",
268 "https://rickandmortyapi.com/api/character/412",
269 "https://rickandmortyapi.com/api/character/413",
270 "https://rickandmortyapi.com/api/character/414",
271 "https://rickandmortyapi.com/api/character/415",
272 "https://rickandmortyapi.com/api/character/416",
273 "https://rickandmortyapi.com/api/character/417",
274 "https://rickandmortyapi.com/api/character/418"
275 ],
276 "url": "https://rickandmortyapi.com/api/episode/8",
277 "created": "2017-11-10T12:56:34.543Z"
278 },
279 {
280 "id": 9,
281 "name": "Something Ricked This Way Comes",
282 "air_date": "March 24, 2014",
283 "episode": "S01E09",
284 "characters": [
285 "https://rickandmortyapi.com/api/character/1",
286 "https://rickandmortyapi.com/api/character/2",
287 "https://rickandmortyapi.com/api/character/3",
288 "https://rickandmortyapi.com/api/character/4",
289 "https://rickandmortyapi.com/api/character/5",
290 "https://rickandmortyapi.com/api/character/88",
291 "https://rickandmortyapi.com/api/character/192",
292 "https://rickandmortyapi.com/api/character/240",
293 "https://rickandmortyapi.com/api/character/243",
294 "https://rickandmortyapi.com/api/character/251",
295 "https://rickandmortyapi.com/api/character/272",
296 "https://rickandmortyapi.com/api/character/307",
297 "https://rickandmortyapi.com/api/character/419",
298 "https://rickandmortyapi.com/api/character/420",
299 "https://rickandmortyapi.com/api/character/421",
300 "https://rickandmortyapi.com/api/character/422"
301 ],
302 "url": "https://rickandmortyapi.com/api/episode/9",
303 "created": "2017-11-10T12:56:34.645Z"
304 },
305 {
306 "id": 10,
307 "name": "Close Rick-counters of the Rick Kind",
308 "air_date": "April 7, 2014",
309 "episode": "S01E10",
310 "characters": [
311 "https://rickandmortyapi.com/api/character/1",
312 "https://rickandmortyapi.com/api/character/2",
313 "https://rickandmortyapi.com/api/character/3",
314 "https://rickandmortyapi.com/api/character/4",
315 "https://rickandmortyapi.com/api/character/5",
316 "https://rickandmortyapi.com/api/character/7",
317 "https://rickandmortyapi.com/api/character/14",
318 "https://rickandmortyapi.com/api/character/15",
319 "https://rickandmortyapi.com/api/character/18",
320 "https://rickandmortyapi.com/api/character/19",
321 "https://rickandmortyapi.com/api/character/21",
322 "https://rickandmortyapi.com/api/character/22",
323 "https://rickandmortyapi.com/api/character/27",
324 "https://rickandmortyapi.com/api/character/39",
325 "https://rickandmortyapi.com/api/character/53",
326 "https://rickandmortyapi.com/api/character/77",
327 "https://rickandmortyapi.com/api/character/78",
328 "https://rickandmortyapi.com/api/character/79",
329 "https://rickandmortyapi.com/api/character/82",
330 "https://rickandmortyapi.com/api/character/83",
331 "https://rickandmortyapi.com/api/character/84",
332 "https://rickandmortyapi.com/api/character/85",
333 "https://rickandmortyapi.com/api/character/86",
334 "https://rickandmortyapi.com/api/character/103",
335 "https://rickandmortyapi.com/api/character/113",
336 "https://rickandmortyapi.com/api/character/118",
337 "https://rickandmortyapi.com/api/character/119",
338 "https://rickandmortyapi.com/api/character/152",
339 "https://rickandmortyapi.com/api/character/164",
340 "https://rickandmortyapi.com/api/character/177",
341 "https://rickandmortyapi.com/api/character/209",
342 "https://rickandmortyapi.com/api/character/215",
343 "https://rickandmortyapi.com/api/character/232",
344 "https://rickandmortyapi.com/api/character/242",
345 "https://rickandmortyapi.com/api/character/274",
346 "https://rickandmortyapi.com/api/character/285",
347 "https://rickandmortyapi.com/api/character/290",
348 "https://rickandmortyapi.com/api/character/294",
349 "https://rickandmortyapi.com/api/character/295",
350 "https://rickandmortyapi.com/api/character/298",
351 "https://rickandmortyapi.com/api/character/299",
352 "https://rickandmortyapi.com/api/character/329",
353 "https://rickandmortyapi.com/api/character/330",
354 "https://rickandmortyapi.com/api/character/339",
355 "https://rickandmortyapi.com/api/character/349",
356 "https://rickandmortyapi.com/api/character/359",
357 "https://rickandmortyapi.com/api/character/381",
358 "https://rickandmortyapi.com/api/character/389",
359 "https://rickandmortyapi.com/api/character/405",
360 "https://rickandmortyapi.com/api/character/424",
361 "https://rickandmortyapi.com/api/character/425",
362 "https://rickandmortyapi.com/api/character/426",
363 "https://rickandmortyapi.com/api/character/427",
364 "https://rickandmortyapi.com/api/character/428",
365 "https://rickandmortyapi.com/api/character/429",
366 "https://rickandmortyapi.com/api/character/430",
367 "https://rickandmortyapi.com/api/character/431",
368 "https://rickandmortyapi.com/api/character/432",
369 "https://rickandmortyapi.com/api/character/433",
370 "https://rickandmortyapi.com/api/character/434",
371 "https://rickandmortyapi.com/api/character/663"
372 ],
373 "url": "https://rickandmortyapi.com/api/episode/10",
374 "created": "2017-11-10T12:56:34.747Z"
375 },
376 {
377 "id": 11,
378 "name": "Ricksy Business",
379 "air_date": "April 14, 2014",
380 "episode": "S01E11",
381 "characters": [
382 "https://rickandmortyapi.com/api/character/1",
383 "https://rickandmortyapi.com/api/character/2",
384 "https://rickandmortyapi.com/api/character/3",
385 "https://rickandmortyapi.com/api/character/4",
386 "https://rickandmortyapi.com/api/character/5",
387 "https://rickandmortyapi.com/api/character/7",
388 "https://rickandmortyapi.com/api/character/35",
389 "https://rickandmortyapi.com/api/character/47",
390 "https://rickandmortyapi.com/api/character/58",
391 "https://rickandmortyapi.com/api/character/88",
392 "https://rickandmortyapi.com/api/character/180",
393 "https://rickandmortyapi.com/api/character/181",
394 "https://rickandmortyapi.com/api/character/210",
395 "https://rickandmortyapi.com/api/character/216",
396 "https://rickandmortyapi.com/api/character/251",
397 "https://rickandmortyapi.com/api/character/282",
398 "https://rickandmortyapi.com/api/character/295",
399 "https://rickandmortyapi.com/api/character/308",
400 "https://rickandmortyapi.com/api/character/326",
401 "https://rickandmortyapi.com/api/character/327",
402 "https://rickandmortyapi.com/api/character/331",
403 "https://rickandmortyapi.com/api/character/333",
404 "https://rickandmortyapi.com/api/character/344",
405 "https://rickandmortyapi.com/api/character/362",
406 "https://rickandmortyapi.com/api/character/389",
407 "https://rickandmortyapi.com/api/character/395",
408 "https://rickandmortyapi.com/api/character/405",
409 "https://rickandmortyapi.com/api/character/423",
410 "https://rickandmortyapi.com/api/character/435",
411 "https://rickandmortyapi.com/api/character/436"
412 ],
413 "url": "https://rickandmortyapi.com/api/episode/11",
414 "created": "2017-11-10T12:56:34.850Z"
415 },
416 {
417 "id": 12,
418 "name": "A Rickle in Time",
419 "air_date": "July 26, 2015",
420 "episode": "S02E01",
421 "characters": [
422 "https://rickandmortyapi.com/api/character/1",
423 "https://rickandmortyapi.com/api/character/2",
424 "https://rickandmortyapi.com/api/character/3",
425 "https://rickandmortyapi.com/api/character/4",
426 "https://rickandmortyapi.com/api/character/5",
427 "https://rickandmortyapi.com/api/character/11",
428 "https://rickandmortyapi.com/api/character/64",
429 "https://rickandmortyapi.com/api/character/237",
430 "https://rickandmortyapi.com/api/character/313",
431 "https://rickandmortyapi.com/api/character/437",
432 "https://rickandmortyapi.com/api/character/438",
433 "https://rickandmortyapi.com/api/character/439",
434 "https://rickandmortyapi.com/api/character/440"
435 ],
436 "url": "https://rickandmortyapi.com/api/episode/12",
437 "created": "2017-11-10T12:56:34.953Z"
438 },
439 {
440 "id": 13,
441 "name": "Mortynight Run",
442 "air_date": "August 2, 2015",
443 "episode": "S02E02",
444 "characters": [
445 "https://rickandmortyapi.com/api/character/1",
446 "https://rickandmortyapi.com/api/character/2",
447 "https://rickandmortyapi.com/api/character/5",
448 "https://rickandmortyapi.com/api/character/23",
449 "https://rickandmortyapi.com/api/character/28",
450 "https://rickandmortyapi.com/api/character/34",
451 "https://rickandmortyapi.com/api/character/106",
452 "https://rickandmortyapi.com/api/character/122",
453 "https://rickandmortyapi.com/api/character/129",
454 "https://rickandmortyapi.com/api/character/131",
455 "https://rickandmortyapi.com/api/character/133",
456 "https://rickandmortyapi.com/api/character/136",
457 "https://rickandmortyapi.com/api/character/174",
458 "https://rickandmortyapi.com/api/character/180",
459 "https://rickandmortyapi.com/api/character/196",
460 "https://rickandmortyapi.com/api/character/207",
461 "https://rickandmortyapi.com/api/character/242",
462 "https://rickandmortyapi.com/api/character/257",
463 "https://rickandmortyapi.com/api/character/282",
464 "https://rickandmortyapi.com/api/character/309",
465 "https://rickandmortyapi.com/api/character/311",
466 "https://rickandmortyapi.com/api/character/362",
467 "https://rickandmortyapi.com/api/character/386",
468 "https://rickandmortyapi.com/api/character/393",
469 "https://rickandmortyapi.com/api/character/436",
470 "https://rickandmortyapi.com/api/character/441",
471 "https://rickandmortyapi.com/api/character/442",
472 "https://rickandmortyapi.com/api/character/443",
473 "https://rickandmortyapi.com/api/character/444",
474 "https://rickandmortyapi.com/api/character/445",
475 "https://rickandmortyapi.com/api/character/446",
476 "https://rickandmortyapi.com/api/character/447",
477 "https://rickandmortyapi.com/api/character/448",
478 "https://rickandmortyapi.com/api/character/449",
479 "https://rickandmortyapi.com/api/character/450",
480 "https://rickandmortyapi.com/api/character/451"
481 ],
482 "url": "https://rickandmortyapi.com/api/episode/13",
483 "created": "2017-11-10T12:56:35.055Z"
484 },
485 {
486 "id": 14,
487 "name": "Auto Erotic Assimilation",
488 "air_date": "August 9, 2015",
489 "episode": "S02E03",
490 "characters": [
491 "https://rickandmortyapi.com/api/character/1",
492 "https://rickandmortyapi.com/api/character/2",
493 "https://rickandmortyapi.com/api/character/3",
494 "https://rickandmortyapi.com/api/character/4",
495 "https://rickandmortyapi.com/api/character/5",
496 "https://rickandmortyapi.com/api/character/36",
497 "https://rickandmortyapi.com/api/character/50",
498 "https://rickandmortyapi.com/api/character/90",
499 "https://rickandmortyapi.com/api/character/188",
500 "https://rickandmortyapi.com/api/character/249",
501 "https://rickandmortyapi.com/api/character/301",
502 "https://rickandmortyapi.com/api/character/336",
503 "https://rickandmortyapi.com/api/character/355",
504 "https://rickandmortyapi.com/api/character/372"
505 ],
506 "url": "https://rickandmortyapi.com/api/episode/14",
507 "created": "2017-11-10T12:56:35.158Z"
508 },
509 {
510 "id": 15,
511 "name": "Total Rickall",
512 "air_date": "August 16, 2015",
513 "episode": "S02E04",
514 "characters": [
515 "https://rickandmortyapi.com/api/character/1",
516 "https://rickandmortyapi.com/api/character/2",
517 "https://rickandmortyapi.com/api/character/3",
518 "https://rickandmortyapi.com/api/character/4",
519 "https://rickandmortyapi.com/api/character/5",
520 "https://rickandmortyapi.com/api/character/16",
521 "https://rickandmortyapi.com/api/character/31",
522 "https://rickandmortyapi.com/api/character/32",
523 "https://rickandmortyapi.com/api/character/76",
524 "https://rickandmortyapi.com/api/character/109",
525 "https://rickandmortyapi.com/api/character/128",
526 "https://rickandmortyapi.com/api/character/141",
527 "https://rickandmortyapi.com/api/character/154",
528 "https://rickandmortyapi.com/api/character/169",
529 "https://rickandmortyapi.com/api/character/236",
530 "https://rickandmortyapi.com/api/character/244",
531 "https://rickandmortyapi.com/api/character/248",
532 "https://rickandmortyapi.com/api/character/259",
533 "https://rickandmortyapi.com/api/character/262",
534 "https://rickandmortyapi.com/api/character/280",
535 "https://rickandmortyapi.com/api/character/324",
536 "https://rickandmortyapi.com/api/character/329",
537 "https://rickandmortyapi.com/api/character/352",
538 "https://rickandmortyapi.com/api/character/391"
539 ],
540 "url": "https://rickandmortyapi.com/api/episode/15",
541 "created": "2017-11-10T12:56:35.261Z"
542 },
543 {
544 "id": 16,
545 "name": "Get Schwifty",
546 "air_date": "August 23, 2015",
547 "episode": "S02E05",
548 "characters": [
549 "https://rickandmortyapi.com/api/character/1",
550 "https://rickandmortyapi.com/api/character/2",
551 "https://rickandmortyapi.com/api/character/3",
552 "https://rickandmortyapi.com/api/character/4",
553 "https://rickandmortyapi.com/api/character/5",
554 "https://rickandmortyapi.com/api/character/24",
555 "https://rickandmortyapi.com/api/character/47",
556 "https://rickandmortyapi.com/api/character/115",
557 "https://rickandmortyapi.com/api/character/124",
558 "https://rickandmortyapi.com/api/character/138",
559 "https://rickandmortyapi.com/api/character/161",
560 "https://rickandmortyapi.com/api/character/162",
561 "https://rickandmortyapi.com/api/character/172",
562 "https://rickandmortyapi.com/api/character/182",
563 "https://rickandmortyapi.com/api/character/199",
564 "https://rickandmortyapi.com/api/character/212",
565 "https://rickandmortyapi.com/api/character/213",
566 "https://rickandmortyapi.com/api/character/240",
567 "https://rickandmortyapi.com/api/character/241",
568 "https://rickandmortyapi.com/api/character/253",
569 "https://rickandmortyapi.com/api/character/255",
570 "https://rickandmortyapi.com/api/character/272",
571 "https://rickandmortyapi.com/api/character/309",
572 "https://rickandmortyapi.com/api/character/329",
573 "https://rickandmortyapi.com/api/character/331",
574 "https://rickandmortyapi.com/api/character/344",
575 "https://rickandmortyapi.com/api/character/346",
576 "https://rickandmortyapi.com/api/character/347",
577 "https://rickandmortyapi.com/api/character/395",
578 "https://rickandmortyapi.com/api/character/452",
579 "https://rickandmortyapi.com/api/character/454"
580 ],
581 "url": "https://rickandmortyapi.com/api/episode/16",
582 "created": "2017-11-10T12:56:35.364Z"
583 },
584 {
585 "id": 17,
586 "name": "The Ricks Must Be Crazy",
587 "air_date": "August 30, 2015",
588 "episode": "S02E06",
589 "characters": [
590 "https://rickandmortyapi.com/api/character/1",
591 "https://rickandmortyapi.com/api/character/2",
592 "https://rickandmortyapi.com/api/character/3",
593 "https://rickandmortyapi.com/api/character/28",
594 "https://rickandmortyapi.com/api/character/34",
595 "https://rickandmortyapi.com/api/character/65",
596 "https://rickandmortyapi.com/api/character/129",
597 "https://rickandmortyapi.com/api/character/159",
598 "https://rickandmortyapi.com/api/character/160",
599 "https://rickandmortyapi.com/api/character/180",
600 "https://rickandmortyapi.com/api/character/181",
601 "https://rickandmortyapi.com/api/character/197",
602 "https://rickandmortyapi.com/api/character/207",
603 "https://rickandmortyapi.com/api/character/240",
604 "https://rickandmortyapi.com/api/character/266",
605 "https://rickandmortyapi.com/api/character/348",
606 "https://rickandmortyapi.com/api/character/364",
607 "https://rickandmortyapi.com/api/character/388"
608 ],
609 "url": "https://rickandmortyapi.com/api/episode/17",
610 "created": "2017-11-10T12:56:35.467Z"
611 },
612 {
613 "id": 18,
614 "name": "Big Trouble in Little Sanchez",
615 "air_date": "September 13, 2015",
616 "episode": "S02E07",
617 "characters": [
618 "https://rickandmortyapi.com/api/character/1",
619 "https://rickandmortyapi.com/api/character/2",
620 "https://rickandmortyapi.com/api/character/3",
621 "https://rickandmortyapi.com/api/character/4",
622 "https://rickandmortyapi.com/api/character/5",
623 "https://rickandmortyapi.com/api/character/40",
624 "https://rickandmortyapi.com/api/character/55",
625 "https://rickandmortyapi.com/api/character/66",
626 "https://rickandmortyapi.com/api/character/131",
627 "https://rickandmortyapi.com/api/character/132",
628 "https://rickandmortyapi.com/api/character/146",
629 "https://rickandmortyapi.com/api/character/148",
630 "https://rickandmortyapi.com/api/character/163",
631 "https://rickandmortyapi.com/api/character/178",
632 "https://rickandmortyapi.com/api/character/180",
633 "https://rickandmortyapi.com/api/character/181",
634 "https://rickandmortyapi.com/api/character/240",
635 "https://rickandmortyapi.com/api/character/272",
636 "https://rickandmortyapi.com/api/character/310",
637 "https://rickandmortyapi.com/api/character/353",
638 "https://rickandmortyapi.com/api/character/354",
639 "https://rickandmortyapi.com/api/character/358",
640 "https://rickandmortyapi.com/api/character/374",
641 "https://rickandmortyapi.com/api/character/386",
642 "https://rickandmortyapi.com/api/character/387",
643 "https://rickandmortyapi.com/api/character/453"
644 ],
645 "url": "https://rickandmortyapi.com/api/episode/18",
646 "created": "2017-11-10T12:56:35.569Z"
647 },
648 {
649 "id": 19,
650 "name": "Interdimensional Cable 2: Tempting Fate",
651 "air_date": "September 20, 2015",
652 "episode": "S02E08",
653 "characters": [
654 "https://rickandmortyapi.com/api/character/1",
655 "https://rickandmortyapi.com/api/character/2",
656 "https://rickandmortyapi.com/api/character/3",
657 "https://rickandmortyapi.com/api/character/4",
658 "https://rickandmortyapi.com/api/character/5",
659 "https://rickandmortyapi.com/api/character/23",
660 "https://rickandmortyapi.com/api/character/35",
661 "https://rickandmortyapi.com/api/character/49",
662 "https://rickandmortyapi.com/api/character/51",
663 "https://rickandmortyapi.com/api/character/105",
664 "https://rickandmortyapi.com/api/character/121",
665 "https://rickandmortyapi.com/api/character/126",
666 "https://rickandmortyapi.com/api/character/133",
667 "https://rickandmortyapi.com/api/character/153",
668 "https://rickandmortyapi.com/api/character/173",
669 "https://rickandmortyapi.com/api/character/199",
670 "https://rickandmortyapi.com/api/character/205",
671 "https://rickandmortyapi.com/api/character/223",
672 "https://rickandmortyapi.com/api/character/224",
673 "https://rickandmortyapi.com/api/character/225",
674 "https://rickandmortyapi.com/api/character/254",
675 "https://rickandmortyapi.com/api/character/260",
676 "https://rickandmortyapi.com/api/character/263",
677 "https://rickandmortyapi.com/api/character/264",
678 "https://rickandmortyapi.com/api/character/275",
679 "https://rickandmortyapi.com/api/character/312",
680 "https://rickandmortyapi.com/api/character/321",
681 "https://rickandmortyapi.com/api/character/334",
682 "https://rickandmortyapi.com/api/character/362",
683 "https://rickandmortyapi.com/api/character/371",
684 "https://rickandmortyapi.com/api/character/383",
685 "https://rickandmortyapi.com/api/character/384",
686 "https://rickandmortyapi.com/api/character/435",
687 "https://rickandmortyapi.com/api/character/454",
688 "https://rickandmortyapi.com/api/character/455",
689 "https://rickandmortyapi.com/api/character/456",
690 "https://rickandmortyapi.com/api/character/457",
691 "https://rickandmortyapi.com/api/character/458",
692 "https://rickandmortyapi.com/api/character/459",
693 "https://rickandmortyapi.com/api/character/460"
694 ],
695 "url": "https://rickandmortyapi.com/api/episode/19",
696 "created": "2017-11-10T12:56:35.669Z"
697 },
698 {
699 "id": 20,
700 "name": "Look Who's Purging Now",
701 "air_date": "September 27, 2015",
702 "episode": "S02E09",
703 "characters": [
704 "https://rickandmortyapi.com/api/character/1",
705 "https://rickandmortyapi.com/api/character/2",
706 "https://rickandmortyapi.com/api/character/3",
707 "https://rickandmortyapi.com/api/character/4",
708 "https://rickandmortyapi.com/api/character/5",
709 "https://rickandmortyapi.com/api/character/26",
710 "https://rickandmortyapi.com/api/character/139",
711 "https://rickandmortyapi.com/api/character/202",
712 "https://rickandmortyapi.com/api/character/273",
713 "https://rickandmortyapi.com/api/character/341"
714 ],
715 "url": "https://rickandmortyapi.com/api/episode/20",
716 "created": "2017-11-10T12:56:35.772Z"
717 }
718 ]
719}
So if we look through all the information we just got we can see that the titles of each episode is in there, but also way more data than we actually need. One thing that we should note though is the information in the info
section, that there are actually 3 pages. It looks like we actually missed that in our GraphQL query, so how do we fix that? Simple, we just ast for that information in our query.
1BASE_URL = 'https://rickandmortyapi.com/graphql'
2
3query = """ {
4 episodes {
5 results {
6 name
7 }
8 info {
9 pages
10 }
11 }
12}
13"""
14
15response = requests.post(BASE_URL, json={'query': query})
16json_object = response.json()
17json_formatted = json.dumps(json_object, indent=4)
18print(json_formatted)
1{
2 "data": {
3 "episodes": {
4 "results": [
5 {
6 "name": "Pilot"
7 },
8 {
9 "name": "Lawnmower Dog"
10 },
11 {
12 "name": "Anatomy Park"
13 },
14 {
15 "name": "M. Night Shaym-Aliens!"
16 },
17 {
18 "name": "Meeseeks and Destroy"
19 },
20 {
21 "name": "Rick Potion #9"
22 },
23 {
24 "name": "Raising Gazorpazorp"
25 },
26 {
27 "name": "Rixty Minutes"
28 },
29 {
30 "name": "Something Ricked This Way Comes"
31 },
32 {
33 "name": "Close Rick-counters of the Rick Kind"
34 },
35 {
36 "name": "Ricksy Business"
37 },
38 {
39 "name": "A Rickle in Time"
40 },
41 {
42 "name": "Mortynight Run"
43 },
44 {
45 "name": "Auto Erotic Assimilation"
46 },
47 {
48 "name": "Total Rickall"
49 },
50 {
51 "name": "Get Schwifty"
52 },
53 {
54 "name": "The Ricks Must Be Crazy"
55 },
56 {
57 "name": "Big Trouble in Little Sanchez"
58 },
59 {
60 "name": "Interdimensional Cable 2: Tempting Fate"
61 },
62 {
63 "name": "Look Who's Purging Now"
64 }
65 ],
66 "info": {
67 "pages": 3
68 }
69 }
70 }
71}
72
So now we know how many pages there are in data set, but how do we actually get them? For the REST API the URL is conveniently given to use.
1response = requests.get('https://rickandmortyapi.com/api/episode?page=2')
2json_object = response.json()
3json_formatted = json.dumps(json_object, indent=4)
4print(json_formatted)
1{
2 "info": {
3 "count": 41,
4 "pages": 3,
5 "next": "https://rickandmortyapi.com/api/episode?page=3",
6 "prev": "https://rickandmortyapi.com/api/episode?page=1"
7 },
8 "results": [
9 {
10 "id": 21,
11 "name": "The Wedding Squanchers",
12 "air_date": "October 4, 2015",
13 "episode": "S02E10",
14 "characters": [
15 "https://rickandmortyapi.com/api/character/1",
16 "https://rickandmortyapi.com/api/character/2",
17 "https://rickandmortyapi.com/api/character/3",
18 "https://rickandmortyapi.com/api/character/4",
19 "https://rickandmortyapi.com/api/character/5",
20 "https://rickandmortyapi.com/api/character/23",
21 "https://rickandmortyapi.com/api/character/47",
22 "https://rickandmortyapi.com/api/character/55",
23 "https://rickandmortyapi.com/api/character/75",
24 "https://rickandmortyapi.com/api/character/102",
25 "https://rickandmortyapi.com/api/character/130",
26 "https://rickandmortyapi.com/api/character/131",
27 "https://rickandmortyapi.com/api/character/133",
28 "https://rickandmortyapi.com/api/character/194",
29 "https://rickandmortyapi.com/api/character/199",
30 "https://rickandmortyapi.com/api/character/203",
31 "https://rickandmortyapi.com/api/character/240",
32 "https://rickandmortyapi.com/api/character/244",
33 "https://rickandmortyapi.com/api/character/256",
34 "https://rickandmortyapi.com/api/character/261",
35 "https://rickandmortyapi.com/api/character/308",
36 "https://rickandmortyapi.com/api/character/309",
37 "https://rickandmortyapi.com/api/character/311",
38 "https://rickandmortyapi.com/api/character/331",
39 "https://rickandmortyapi.com/api/character/344",
40 "https://rickandmortyapi.com/api/character/358",
41 "https://rickandmortyapi.com/api/character/362",
42 "https://rickandmortyapi.com/api/character/379",
43 "https://rickandmortyapi.com/api/character/454"
44 ],
45 "url": "https://rickandmortyapi.com/api/episode/21",
46 "created": "2017-11-10T12:56:35.875Z"
47 },
48 {
49 "id": 22,
50 "name": "The Rickshank Rickdemption",
51 "air_date": "April 1, 2017",
52 "episode": "S03E01",
53 "characters": [
54 "https://rickandmortyapi.com/api/character/1",
55 "https://rickandmortyapi.com/api/character/2",
56 "https://rickandmortyapi.com/api/character/3",
57 "https://rickandmortyapi.com/api/character/4",
58 "https://rickandmortyapi.com/api/character/5",
59 "https://rickandmortyapi.com/api/character/21",
60 "https://rickandmortyapi.com/api/character/22",
61 "https://rickandmortyapi.com/api/character/38",
62 "https://rickandmortyapi.com/api/character/42",
63 "https://rickandmortyapi.com/api/character/47",
64 "https://rickandmortyapi.com/api/character/48",
65 "https://rickandmortyapi.com/api/character/57",
66 "https://rickandmortyapi.com/api/character/69",
67 "https://rickandmortyapi.com/api/character/71",
68 "https://rickandmortyapi.com/api/character/86",
69 "https://rickandmortyapi.com/api/character/94",
70 "https://rickandmortyapi.com/api/character/95",
71 "https://rickandmortyapi.com/api/character/103",
72 "https://rickandmortyapi.com/api/character/150",
73 "https://rickandmortyapi.com/api/character/152",
74 "https://rickandmortyapi.com/api/character/175",
75 "https://rickandmortyapi.com/api/character/200",
76 "https://rickandmortyapi.com/api/character/215",
77 "https://rickandmortyapi.com/api/character/231",
78 "https://rickandmortyapi.com/api/character/240",
79 "https://rickandmortyapi.com/api/character/274",
80 "https://rickandmortyapi.com/api/character/285",
81 "https://rickandmortyapi.com/api/character/286",
82 "https://rickandmortyapi.com/api/character/294",
83 "https://rickandmortyapi.com/api/character/295",
84 "https://rickandmortyapi.com/api/character/330",
85 "https://rickandmortyapi.com/api/character/338",
86 "https://rickandmortyapi.com/api/character/344",
87 "https://rickandmortyapi.com/api/character/378",
88 "https://rickandmortyapi.com/api/character/380",
89 "https://rickandmortyapi.com/api/character/385",
90 "https://rickandmortyapi.com/api/character/389",
91 "https://rickandmortyapi.com/api/character/461",
92 "https://rickandmortyapi.com/api/character/462",
93 "https://rickandmortyapi.com/api/character/463",
94 "https://rickandmortyapi.com/api/character/464",
95 "https://rickandmortyapi.com/api/character/465",
96 "https://rickandmortyapi.com/api/character/466",
97 "https://rickandmortyapi.com/api/character/592"
98 ],
99 "url": "https://rickandmortyapi.com/api/episode/22",
100 "created": "2017-11-10T12:56:35.983Z"
101 },
102 {
103 "id": 23,
104 "name": "Rickmancing the Stone",
105 "air_date": "July 30, 2017",
106 "episode": "S03E02",
107 "characters": [
108 "https://rickandmortyapi.com/api/character/1",
109 "https://rickandmortyapi.com/api/character/2",
110 "https://rickandmortyapi.com/api/character/3",
111 "https://rickandmortyapi.com/api/character/4",
112 "https://rickandmortyapi.com/api/character/5",
113 "https://rickandmortyapi.com/api/character/25",
114 "https://rickandmortyapi.com/api/character/52",
115 "https://rickandmortyapi.com/api/character/68",
116 "https://rickandmortyapi.com/api/character/110",
117 "https://rickandmortyapi.com/api/character/111",
118 "https://rickandmortyapi.com/api/character/140",
119 "https://rickandmortyapi.com/api/character/156",
120 "https://rickandmortyapi.com/api/character/217",
121 "https://rickandmortyapi.com/api/character/218",
122 "https://rickandmortyapi.com/api/character/219",
123 "https://rickandmortyapi.com/api/character/228",
124 "https://rickandmortyapi.com/api/character/323",
125 "https://rickandmortyapi.com/api/character/342"
126 ],
127 "url": "https://rickandmortyapi.com/api/episode/23",
128 "created": "2017-11-10T12:56:36.100Z"
129 },
130 {
131 "id": 24,
132 "name": "Pickle Rick",
133 "air_date": "August 6, 2017",
134 "episode": "S03E03",
135 "characters": [
136 "https://rickandmortyapi.com/api/character/1",
137 "https://rickandmortyapi.com/api/character/2",
138 "https://rickandmortyapi.com/api/character/3",
139 "https://rickandmortyapi.com/api/character/4",
140 "https://rickandmortyapi.com/api/character/9",
141 "https://rickandmortyapi.com/api/character/70",
142 "https://rickandmortyapi.com/api/character/107",
143 "https://rickandmortyapi.com/api/character/167",
144 "https://rickandmortyapi.com/api/character/171",
145 "https://rickandmortyapi.com/api/character/189",
146 "https://rickandmortyapi.com/api/character/240",
147 "https://rickandmortyapi.com/api/character/265",
148 "https://rickandmortyapi.com/api/character/272",
149 "https://rickandmortyapi.com/api/character/276",
150 "https://rickandmortyapi.com/api/character/329"
151 ],
152 "url": "https://rickandmortyapi.com/api/episode/24",
153 "created": "2017-11-10T12:56:36.206Z"
154 },
155 {
156 "id": 25,
157 "name": "Vindicators 3: The Return of Worldender",
158 "air_date": "August 13, 2017",
159 "episode": "S03E04",
160 "characters": [
161 "https://rickandmortyapi.com/api/character/1",
162 "https://rickandmortyapi.com/api/character/2",
163 "https://rickandmortyapi.com/api/character/3",
164 "https://rickandmortyapi.com/api/character/4",
165 "https://rickandmortyapi.com/api/character/10",
166 "https://rickandmortyapi.com/api/character/23",
167 "https://rickandmortyapi.com/api/character/35",
168 "https://rickandmortyapi.com/api/character/60",
169 "https://rickandmortyapi.com/api/character/81",
170 "https://rickandmortyapi.com/api/character/88",
171 "https://rickandmortyapi.com/api/character/93",
172 "https://rickandmortyapi.com/api/character/104",
173 "https://rickandmortyapi.com/api/character/125",
174 "https://rickandmortyapi.com/api/character/181",
175 "https://rickandmortyapi.com/api/character/198",
176 "https://rickandmortyapi.com/api/character/208",
177 "https://rickandmortyapi.com/api/character/216",
178 "https://rickandmortyapi.com/api/character/226",
179 "https://rickandmortyapi.com/api/character/251",
180 "https://rickandmortyapi.com/api/character/252",
181 "https://rickandmortyapi.com/api/character/282",
182 "https://rickandmortyapi.com/api/character/309",
183 "https://rickandmortyapi.com/api/character/311",
184 "https://rickandmortyapi.com/api/character/333",
185 "https://rickandmortyapi.com/api/character/340",
186 "https://rickandmortyapi.com/api/character/362",
187 "https://rickandmortyapi.com/api/character/375",
188 "https://rickandmortyapi.com/api/character/382",
189 "https://rickandmortyapi.com/api/character/395",
190 "https://rickandmortyapi.com/api/character/435"
191 ],
192 "url": "https://rickandmortyapi.com/api/episode/25",
193 "created": "2017-11-10T12:56:36.310Z"
194 },
195 {
196 "id": 26,
197 "name": "The Whirly Dirly Conspiracy",
198 "air_date": "August 20, 2017",
199 "episode": "S03E05",
200 "characters": [
201 "https://rickandmortyapi.com/api/character/1",
202 "https://rickandmortyapi.com/api/character/2",
203 "https://rickandmortyapi.com/api/character/3",
204 "https://rickandmortyapi.com/api/character/4",
205 "https://rickandmortyapi.com/api/character/5",
206 "https://rickandmortyapi.com/api/character/23",
207 "https://rickandmortyapi.com/api/character/47",
208 "https://rickandmortyapi.com/api/character/115",
209 "https://rickandmortyapi.com/api/character/137",
210 "https://rickandmortyapi.com/api/character/142",
211 "https://rickandmortyapi.com/api/character/180",
212 "https://rickandmortyapi.com/api/character/204",
213 "https://rickandmortyapi.com/api/character/296",
214 "https://rickandmortyapi.com/api/character/297",
215 "https://rickandmortyapi.com/api/character/319",
216 "https://rickandmortyapi.com/api/character/320",
217 "https://rickandmortyapi.com/api/character/365",
218 "https://rickandmortyapi.com/api/character/369",
219 "https://rickandmortyapi.com/api/character/467",
220 "https://rickandmortyapi.com/api/character/468",
221 "https://rickandmortyapi.com/api/character/469"
222 ],
223 "url": "https://rickandmortyapi.com/api/episode/26",
224 "created": "2017-11-10T12:56:36.413Z"
225 },
226 {
227 "id": 27,
228 "name": "Rest and Ricklaxation",
229 "air_date": "August 27, 2017",
230 "episode": "S03E06",
231 "characters": [
232 "https://rickandmortyapi.com/api/character/1",
233 "https://rickandmortyapi.com/api/character/2",
234 "https://rickandmortyapi.com/api/character/3",
235 "https://rickandmortyapi.com/api/character/4",
236 "https://rickandmortyapi.com/api/character/6",
237 "https://rickandmortyapi.com/api/character/124",
238 "https://rickandmortyapi.com/api/character/170",
239 "https://rickandmortyapi.com/api/character/180",
240 "https://rickandmortyapi.com/api/character/181",
241 "https://rickandmortyapi.com/api/character/227",
242 "https://rickandmortyapi.com/api/character/240",
243 "https://rickandmortyapi.com/api/character/246",
244 "https://rickandmortyapi.com/api/character/272",
245 "https://rickandmortyapi.com/api/character/332",
246 "https://rickandmortyapi.com/api/character/360",
247 "https://rickandmortyapi.com/api/character/361",
248 "https://rickandmortyapi.com/api/character/365",
249 "https://rickandmortyapi.com/api/character/470",
250 "https://rickandmortyapi.com/api/character/471"
251 ],
252 "url": "https://rickandmortyapi.com/api/episode/27",
253 "created": "2017-11-10T12:56:36.515Z"
254 },
255 {
256 "id": 28,
257 "name": "The Ricklantis Mixup",
258 "air_date": "September 10, 2017",
259 "episode": "S03E07",
260 "characters": [
261 "https://rickandmortyapi.com/api/character/1",
262 "https://rickandmortyapi.com/api/character/2",
263 "https://rickandmortyapi.com/api/character/4",
264 "https://rickandmortyapi.com/api/character/8",
265 "https://rickandmortyapi.com/api/character/18",
266 "https://rickandmortyapi.com/api/character/22",
267 "https://rickandmortyapi.com/api/character/27",
268 "https://rickandmortyapi.com/api/character/43",
269 "https://rickandmortyapi.com/api/character/44",
270 "https://rickandmortyapi.com/api/character/48",
271 "https://rickandmortyapi.com/api/character/56",
272 "https://rickandmortyapi.com/api/character/61",
273 "https://rickandmortyapi.com/api/character/72",
274 "https://rickandmortyapi.com/api/character/73",
275 "https://rickandmortyapi.com/api/character/74",
276 "https://rickandmortyapi.com/api/character/78",
277 "https://rickandmortyapi.com/api/character/85",
278 "https://rickandmortyapi.com/api/character/86",
279 "https://rickandmortyapi.com/api/character/118",
280 "https://rickandmortyapi.com/api/character/123",
281 "https://rickandmortyapi.com/api/character/135",
282 "https://rickandmortyapi.com/api/character/143",
283 "https://rickandmortyapi.com/api/character/165",
284 "https://rickandmortyapi.com/api/character/180",
285 "https://rickandmortyapi.com/api/character/187",
286 "https://rickandmortyapi.com/api/character/206",
287 "https://rickandmortyapi.com/api/character/220",
288 "https://rickandmortyapi.com/api/character/229",
289 "https://rickandmortyapi.com/api/character/233",
290 "https://rickandmortyapi.com/api/character/235",
291 "https://rickandmortyapi.com/api/character/267",
292 "https://rickandmortyapi.com/api/character/278",
293 "https://rickandmortyapi.com/api/character/281",
294 "https://rickandmortyapi.com/api/character/283",
295 "https://rickandmortyapi.com/api/character/284",
296 "https://rickandmortyapi.com/api/character/287",
297 "https://rickandmortyapi.com/api/character/288",
298 "https://rickandmortyapi.com/api/character/289",
299 "https://rickandmortyapi.com/api/character/291",
300 "https://rickandmortyapi.com/api/character/292",
301 "https://rickandmortyapi.com/api/character/322",
302 "https://rickandmortyapi.com/api/character/325",
303 "https://rickandmortyapi.com/api/character/328",
304 "https://rickandmortyapi.com/api/character/345",
305 "https://rickandmortyapi.com/api/character/366",
306 "https://rickandmortyapi.com/api/character/367",
307 "https://rickandmortyapi.com/api/character/392",
308 "https://rickandmortyapi.com/api/character/472",
309 "https://rickandmortyapi.com/api/character/473",
310 "https://rickandmortyapi.com/api/character/474",
311 "https://rickandmortyapi.com/api/character/475",
312 "https://rickandmortyapi.com/api/character/476",
313 "https://rickandmortyapi.com/api/character/477",
314 "https://rickandmortyapi.com/api/character/478",
315 "https://rickandmortyapi.com/api/character/479",
316 "https://rickandmortyapi.com/api/character/480",
317 "https://rickandmortyapi.com/api/character/481",
318 "https://rickandmortyapi.com/api/character/482",
319 "https://rickandmortyapi.com/api/character/483",
320 "https://rickandmortyapi.com/api/character/484",
321 "https://rickandmortyapi.com/api/character/485",
322 "https://rickandmortyapi.com/api/character/486",
323 "https://rickandmortyapi.com/api/character/487",
324 "https://rickandmortyapi.com/api/character/488",
325 "https://rickandmortyapi.com/api/character/489"
326 ],
327 "url": "https://rickandmortyapi.com/api/episode/28",
328 "created": "2017-11-10T12:56:36.618Z"
329 },
330 {
331 "id": 29,
332 "name": "Morty's Mind Blowers",
333 "air_date": "September 17, 2017",
334 "episode": "S03E08",
335 "characters": [
336 "https://rickandmortyapi.com/api/character/1",
337 "https://rickandmortyapi.com/api/character/2",
338 "https://rickandmortyapi.com/api/character/3",
339 "https://rickandmortyapi.com/api/character/4",
340 "https://rickandmortyapi.com/api/character/5",
341 "https://rickandmortyapi.com/api/character/33",
342 "https://rickandmortyapi.com/api/character/67",
343 "https://rickandmortyapi.com/api/character/147",
344 "https://rickandmortyapi.com/api/character/149",
345 "https://rickandmortyapi.com/api/character/180",
346 "https://rickandmortyapi.com/api/character/242",
347 "https://rickandmortyapi.com/api/character/244",
348 "https://rickandmortyapi.com/api/character/251",
349 "https://rickandmortyapi.com/api/character/272",
350 "https://rickandmortyapi.com/api/character/329",
351 "https://rickandmortyapi.com/api/character/368",
352 "https://rickandmortyapi.com/api/character/377",
353 "https://rickandmortyapi.com/api/character/390",
354 "https://rickandmortyapi.com/api/character/490",
355 "https://rickandmortyapi.com/api/character/491"
356 ],
357 "url": "https://rickandmortyapi.com/api/episode/29",
358 "created": "2017-11-10T12:56:36.726Z"
359 },
360 {
361 "id": 30,
362 "name": "The ABC's of Beth",
363 "air_date": "September 24, 2017",
364 "episode": "S03E09",
365 "characters": [
366 "https://rickandmortyapi.com/api/character/1",
367 "https://rickandmortyapi.com/api/character/2",
368 "https://rickandmortyapi.com/api/character/3",
369 "https://rickandmortyapi.com/api/character/4",
370 "https://rickandmortyapi.com/api/character/5",
371 "https://rickandmortyapi.com/api/character/58",
372 "https://rickandmortyapi.com/api/character/180",
373 "https://rickandmortyapi.com/api/character/185",
374 "https://rickandmortyapi.com/api/character/190",
375 "https://rickandmortyapi.com/api/character/240",
376 "https://rickandmortyapi.com/api/character/244",
377 "https://rickandmortyapi.com/api/character/245",
378 "https://rickandmortyapi.com/api/character/249",
379 "https://rickandmortyapi.com/api/character/329",
380 "https://rickandmortyapi.com/api/character/350",
381 "https://rickandmortyapi.com/api/character/357",
382 "https://rickandmortyapi.com/api/character/363",
383 "https://rickandmortyapi.com/api/character/492"
384 ],
385 "url": "https://rickandmortyapi.com/api/episode/30",
386 "created": "2017-11-10T12:56:36.828Z"
387 },
388 {
389 "id": 31,
390 "name": "The Rickchurian Mortydate",
391 "air_date": "October 1, 2017",
392 "episode": "S03E10",
393 "characters": [
394 "https://rickandmortyapi.com/api/character/1",
395 "https://rickandmortyapi.com/api/character/2",
396 "https://rickandmortyapi.com/api/character/3",
397 "https://rickandmortyapi.com/api/character/4",
398 "https://rickandmortyapi.com/api/character/5",
399 "https://rickandmortyapi.com/api/character/13",
400 "https://rickandmortyapi.com/api/character/30",
401 "https://rickandmortyapi.com/api/character/166",
402 "https://rickandmortyapi.com/api/character/244",
403 "https://rickandmortyapi.com/api/character/247",
404 "https://rickandmortyapi.com/api/character/269",
405 "https://rickandmortyapi.com/api/character/335",
406 "https://rickandmortyapi.com/api/character/347",
407 "https://rickandmortyapi.com/api/character/493"
408 ],
409 "url": "https://rickandmortyapi.com/api/episode/31",
410 "created": "2017-11-10T12:56:36.929Z"
411 },
412 {
413 "id": 32,
414 "name": "Edge of Tomorty: Rick, Die, Rickpeat",
415 "air_date": "November 10, 2019",
416 "episode": "S04E01",
417 "characters": [
418 "https://rickandmortyapi.com/api/character/1",
419 "https://rickandmortyapi.com/api/character/2",
420 "https://rickandmortyapi.com/api/character/3",
421 "https://rickandmortyapi.com/api/character/4",
422 "https://rickandmortyapi.com/api/character/5",
423 "https://rickandmortyapi.com/api/character/180",
424 "https://rickandmortyapi.com/api/character/242",
425 "https://rickandmortyapi.com/api/character/494",
426 "https://rickandmortyapi.com/api/character/495",
427 "https://rickandmortyapi.com/api/character/496",
428 "https://rickandmortyapi.com/api/character/497",
429 "https://rickandmortyapi.com/api/character/498",
430 "https://rickandmortyapi.com/api/character/499",
431 "https://rickandmortyapi.com/api/character/500",
432 "https://rickandmortyapi.com/api/character/501",
433 "https://rickandmortyapi.com/api/character/502",
434 "https://rickandmortyapi.com/api/character/503",
435 "https://rickandmortyapi.com/api/character/504",
436 "https://rickandmortyapi.com/api/character/505",
437 "https://rickandmortyapi.com/api/character/506",
438 "https://rickandmortyapi.com/api/character/507",
439 "https://rickandmortyapi.com/api/character/508",
440 "https://rickandmortyapi.com/api/character/509",
441 "https://rickandmortyapi.com/api/character/510",
442 "https://rickandmortyapi.com/api/character/511",
443 "https://rickandmortyapi.com/api/character/512",
444 "https://rickandmortyapi.com/api/character/513",
445 "https://rickandmortyapi.com/api/character/514",
446 "https://rickandmortyapi.com/api/character/515",
447 "https://rickandmortyapi.com/api/character/516",
448 "https://rickandmortyapi.com/api/character/517",
449 "https://rickandmortyapi.com/api/character/518",
450 "https://rickandmortyapi.com/api/character/519",
451 "https://rickandmortyapi.com/api/character/520",
452 "https://rickandmortyapi.com/api/character/521",
453 "https://rickandmortyapi.com/api/character/522",
454 "https://rickandmortyapi.com/api/character/523",
455 "https://rickandmortyapi.com/api/character/524"
456 ],
457 "url": "https://rickandmortyapi.com/api/episode/32",
458 "created": "2020-04-30T06:52:04.495Z"
459 },
460 {
461 "id": 33,
462 "name": "The Old Man and the Seat",
463 "air_date": "November 17, 2019",
464 "episode": "S04E02",
465 "characters": [
466 "https://rickandmortyapi.com/api/character/1",
467 "https://rickandmortyapi.com/api/character/2",
468 "https://rickandmortyapi.com/api/character/3",
469 "https://rickandmortyapi.com/api/character/4",
470 "https://rickandmortyapi.com/api/character/5",
471 "https://rickandmortyapi.com/api/character/525",
472 "https://rickandmortyapi.com/api/character/526",
473 "https://rickandmortyapi.com/api/character/527",
474 "https://rickandmortyapi.com/api/character/528",
475 "https://rickandmortyapi.com/api/character/529",
476 "https://rickandmortyapi.com/api/character/530",
477 "https://rickandmortyapi.com/api/character/531",
478 "https://rickandmortyapi.com/api/character/532",
479 "https://rickandmortyapi.com/api/character/533",
480 "https://rickandmortyapi.com/api/character/534",
481 "https://rickandmortyapi.com/api/character/535",
482 "https://rickandmortyapi.com/api/character/536",
483 "https://rickandmortyapi.com/api/character/537",
484 "https://rickandmortyapi.com/api/character/538",
485 "https://rickandmortyapi.com/api/character/539",
486 "https://rickandmortyapi.com/api/character/540",
487 "https://rickandmortyapi.com/api/character/541",
488 "https://rickandmortyapi.com/api/character/542",
489 "https://rickandmortyapi.com/api/character/543"
490 ],
491 "url": "https://rickandmortyapi.com/api/episode/33",
492 "created": "2020-04-30T06:52:04.498Z"
493 },
494 {
495 "id": 34,
496 "name": "One Crew Over the Crewcoo's Morty",
497 "air_date": "November 24, 2019",
498 "episode": "S04E03",
499 "characters": [
500 "https://rickandmortyapi.com/api/character/1",
501 "https://rickandmortyapi.com/api/character/2",
502 "https://rickandmortyapi.com/api/character/3",
503 "https://rickandmortyapi.com/api/character/4",
504 "https://rickandmortyapi.com/api/character/244",
505 "https://rickandmortyapi.com/api/character/544",
506 "https://rickandmortyapi.com/api/character/545",
507 "https://rickandmortyapi.com/api/character/546",
508 "https://rickandmortyapi.com/api/character/547",
509 "https://rickandmortyapi.com/api/character/548",
510 "https://rickandmortyapi.com/api/character/549",
511 "https://rickandmortyapi.com/api/character/550",
512 "https://rickandmortyapi.com/api/character/551",
513 "https://rickandmortyapi.com/api/character/552",
514 "https://rickandmortyapi.com/api/character/553",
515 "https://rickandmortyapi.com/api/character/554",
516 "https://rickandmortyapi.com/api/character/555",
517 "https://rickandmortyapi.com/api/character/556",
518 "https://rickandmortyapi.com/api/character/557",
519 "https://rickandmortyapi.com/api/character/558",
520 "https://rickandmortyapi.com/api/character/559",
521 "https://rickandmortyapi.com/api/character/560",
522 "https://rickandmortyapi.com/api/character/561"
523 ],
524 "url": "https://rickandmortyapi.com/api/episode/34",
525 "created": "2020-04-30T06:52:04.498Z"
526 },
527 {
528 "id": 35,
529 "name": "Claw and Hoarder: Special Ricktim's Morty",
530 "air_date": "December 8, 2019",
531 "episode": "S04E04",
532 "characters": [
533 "https://rickandmortyapi.com/api/character/1",
534 "https://rickandmortyapi.com/api/character/2",
535 "https://rickandmortyapi.com/api/character/3",
536 "https://rickandmortyapi.com/api/character/4",
537 "https://rickandmortyapi.com/api/character/5",
538 "https://rickandmortyapi.com/api/character/562",
539 "https://rickandmortyapi.com/api/character/563",
540 "https://rickandmortyapi.com/api/character/564",
541 "https://rickandmortyapi.com/api/character/565",
542 "https://rickandmortyapi.com/api/character/566",
543 "https://rickandmortyapi.com/api/character/567",
544 "https://rickandmortyapi.com/api/character/568",
545 "https://rickandmortyapi.com/api/character/569",
546 "https://rickandmortyapi.com/api/character/570"
547 ],
548 "url": "https://rickandmortyapi.com/api/episode/35",
549 "created": "2020-04-30T06:52:04.498Z"
550 },
551 {
552 "id": 36,
553 "name": "Rattlestar Ricklactica",
554 "air_date": "December 15, 2019",
555 "episode": "S04E05",
556 "characters": [
557 "https://rickandmortyapi.com/api/character/1",
558 "https://rickandmortyapi.com/api/character/2",
559 "https://rickandmortyapi.com/api/character/3",
560 "https://rickandmortyapi.com/api/character/4",
561 "https://rickandmortyapi.com/api/character/5",
562 "https://rickandmortyapi.com/api/character/251",
563 "https://rickandmortyapi.com/api/character/313",
564 "https://rickandmortyapi.com/api/character/365",
565 "https://rickandmortyapi.com/api/character/571",
566 "https://rickandmortyapi.com/api/character/572",
567 "https://rickandmortyapi.com/api/character/573",
568 "https://rickandmortyapi.com/api/character/574",
569 "https://rickandmortyapi.com/api/character/575",
570 "https://rickandmortyapi.com/api/character/576",
571 "https://rickandmortyapi.com/api/character/577",
572 "https://rickandmortyapi.com/api/character/578",
573 "https://rickandmortyapi.com/api/character/579",
574 "https://rickandmortyapi.com/api/character/580",
575 "https://rickandmortyapi.com/api/character/581",
576 "https://rickandmortyapi.com/api/character/582",
577 "https://rickandmortyapi.com/api/character/583",
578 "https://rickandmortyapi.com/api/character/584",
579 "https://rickandmortyapi.com/api/character/585",
580 "https://rickandmortyapi.com/api/character/586",
581 "https://rickandmortyapi.com/api/character/587",
582 "https://rickandmortyapi.com/api/character/588",
583 "https://rickandmortyapi.com/api/character/589",
584 "https://rickandmortyapi.com/api/character/590",
585 "https://rickandmortyapi.com/api/character/591"
586 ],
587 "url": "https://rickandmortyapi.com/api/episode/36",
588 "created": "2020-04-30T06:52:04.499Z"
589 },
590 {
591 "id": 37,
592 "name": "Never Ricking Morty",
593 "air_date": "May 3, 2020",
594 "episode": "S04E06",
595 "characters": [
596 "https://rickandmortyapi.com/api/character/593",
597 "https://rickandmortyapi.com/api/character/594",
598 "https://rickandmortyapi.com/api/character/595",
599 "https://rickandmortyapi.com/api/character/596",
600 "https://rickandmortyapi.com/api/character/597",
601 "https://rickandmortyapi.com/api/character/598",
602 "https://rickandmortyapi.com/api/character/599",
603 "https://rickandmortyapi.com/api/character/600",
604 "https://rickandmortyapi.com/api/character/601",
605 "https://rickandmortyapi.com/api/character/602",
606 "https://rickandmortyapi.com/api/character/603",
607 "https://rickandmortyapi.com/api/character/604",
608 "https://rickandmortyapi.com/api/character/605",
609 "https://rickandmortyapi.com/api/character/606",
610 "https://rickandmortyapi.com/api/character/607",
611 "https://rickandmortyapi.com/api/character/608",
612 "https://rickandmortyapi.com/api/character/609",
613 "https://rickandmortyapi.com/api/character/610",
614 "https://rickandmortyapi.com/api/character/611",
615 "https://rickandmortyapi.com/api/character/612",
616 "https://rickandmortyapi.com/api/character/613",
617 "https://rickandmortyapi.com/api/character/614",
618 "https://rickandmortyapi.com/api/character/615",
619 "https://rickandmortyapi.com/api/character/616",
620 "https://rickandmortyapi.com/api/character/617",
621 "https://rickandmortyapi.com/api/character/618",
622 "https://rickandmortyapi.com/api/character/619",
623 "https://rickandmortyapi.com/api/character/620",
624 "https://rickandmortyapi.com/api/character/621",
625 "https://rickandmortyapi.com/api/character/622",
626 "https://rickandmortyapi.com/api/character/623",
627 "https://rickandmortyapi.com/api/character/624",
628 "https://rickandmortyapi.com/api/character/625",
629 "https://rickandmortyapi.com/api/character/626",
630 "https://rickandmortyapi.com/api/character/627",
631 "https://rickandmortyapi.com/api/character/628",
632 "https://rickandmortyapi.com/api/character/629",
633 "https://rickandmortyapi.com/api/character/630",
634 "https://rickandmortyapi.com/api/character/631",
635 "https://rickandmortyapi.com/api/character/632",
636 "https://rickandmortyapi.com/api/character/633",
637 "https://rickandmortyapi.com/api/character/634",
638 "https://rickandmortyapi.com/api/character/635",
639 "https://rickandmortyapi.com/api/character/636",
640 "https://rickandmortyapi.com/api/character/637",
641 "https://rickandmortyapi.com/api/character/638",
642 "https://rickandmortyapi.com/api/character/639",
643 "https://rickandmortyapi.com/api/character/1",
644 "https://rickandmortyapi.com/api/character/2"
645 ],
646 "url": "https://rickandmortyapi.com/api/episode/37",
647 "created": "2020-08-06T05:44:21.422Z"
648 },
649 {
650 "id": 38,
651 "name": "Promortyus",
652 "air_date": "May 10, 2020",
653 "episode": "S04E07",
654 "characters": [
655 "https://rickandmortyapi.com/api/character/640",
656 "https://rickandmortyapi.com/api/character/641",
657 "https://rickandmortyapi.com/api/character/642",
658 "https://rickandmortyapi.com/api/character/643",
659 "https://rickandmortyapi.com/api/character/644",
660 "https://rickandmortyapi.com/api/character/645",
661 "https://rickandmortyapi.com/api/character/646",
662 "https://rickandmortyapi.com/api/character/647",
663 "https://rickandmortyapi.com/api/character/1",
664 "https://rickandmortyapi.com/api/character/2",
665 "https://rickandmortyapi.com/api/character/3",
666 "https://rickandmortyapi.com/api/character/4",
667 "https://rickandmortyapi.com/api/character/5",
668 "https://rickandmortyapi.com/api/character/365"
669 ],
670 "url": "https://rickandmortyapi.com/api/episode/38",
671 "created": "2020-08-06T05:49:40.563Z"
672 },
673 {
674 "id": 39,
675 "name": "The Vat of Acid Episode",
676 "air_date": "May 17, 2020",
677 "episode": "S04E08",
678 "characters": [
679 "https://rickandmortyapi.com/api/character/1",
680 "https://rickandmortyapi.com/api/character/2",
681 "https://rickandmortyapi.com/api/character/4",
682 "https://rickandmortyapi.com/api/character/3",
683 "https://rickandmortyapi.com/api/character/5",
684 "https://rickandmortyapi.com/api/character/240",
685 "https://rickandmortyapi.com/api/character/180",
686 "https://rickandmortyapi.com/api/character/648",
687 "https://rickandmortyapi.com/api/character/649",
688 "https://rickandmortyapi.com/api/character/650",
689 "https://rickandmortyapi.com/api/character/651",
690 "https://rickandmortyapi.com/api/character/652",
691 "https://rickandmortyapi.com/api/character/653",
692 "https://rickandmortyapi.com/api/character/654",
693 "https://rickandmortyapi.com/api/character/655",
694 "https://rickandmortyapi.com/api/character/656",
695 "https://rickandmortyapi.com/api/character/657",
696 "https://rickandmortyapi.com/api/character/658",
697 "https://rickandmortyapi.com/api/character/659",
698 "https://rickandmortyapi.com/api/character/660",
699 "https://rickandmortyapi.com/api/character/661"
700 ],
701 "url": "https://rickandmortyapi.com/api/episode/39",
702 "created": "2020-08-06T05:51:07.419Z"
703 },
704 {
705 "id": 40,
706 "name": "Childrick of Mort",
707 "air_date": "May 24, 2020",
708 "episode": "S04E09",
709 "characters": [
710 "https://rickandmortyapi.com/api/character/1",
711 "https://rickandmortyapi.com/api/character/2",
712 "https://rickandmortyapi.com/api/character/3",
713 "https://rickandmortyapi.com/api/character/4",
714 "https://rickandmortyapi.com/api/character/5",
715 "https://rickandmortyapi.com/api/character/662",
716 "https://rickandmortyapi.com/api/character/663",
717 "https://rickandmortyapi.com/api/character/664",
718 "https://rickandmortyapi.com/api/character/665",
719 "https://rickandmortyapi.com/api/character/666"
720 ],
721 "url": "https://rickandmortyapi.com/api/episode/40",
722 "created": "2020-08-06T05:51:25.458Z"
723 }
724 ]
725}
But what do we do for the GraphQL query? It is actually even easier than the change for the REST API. We actually use the exact same query, but we just put in an argument for the page that we want by changing the first line of our query to episodes(page: 2) {
.
1BASE_URL = 'https://rickandmortyapi.com/graphql'
2
3query = """ {
4 episodes(page: 2) {
5 results {
6 name
7 }
8 info {
9 pages
10 }
11 }
12}
13"""
14
15response = requests.post(BASE_URL, json={'query': query})
16json_object = response.json()
17json_formatted = json.dumps(json_object, indent=4)
18print(json_formatted)
1{
2 "data": {
3 "episodes": {
4 "results": [
5 {
6 "name": "The Wedding Squanchers"
7 },
8 {
9 "name": "The Rickshank Rickdemption"
10 },
11 {
12 "name": "Rickmancing the Stone"
13 },
14 {
15 "name": "Pickle Rick"
16 },
17 {
18 "name": "Vindicators 3: The Return of Worldender"
19 },
20 {
21 "name": "The Whirly Dirly Conspiracy"
22 },
23 {
24 "name": "Rest and Ricklaxation"
25 },
26 {
27 "name": "The Ricklantis Mixup"
28 },
29 {
30 "name": "Morty's Mind Blowers"
31 },
32 {
33 "name": "The ABC's of Beth"
34 },
35 {
36 "name": "The Rickchurian Mortydate"
37 },
38 {
39 "name": "Edge of Tomorty: Rick, Die, Rickpeat"
40 },
41 {
42 "name": "The Old Man and the Seat"
43 },
44 {
45 "name": "One Crew Over the Crewcoo's Morty"
46 },
47 {
48 "name": "Claw and Hoarder: Special Ricktim's Morty"
49 },
50 {
51 "name": "Rattlestar Ricklactica"
52 },
53 {
54 "name": "Never Ricking Morty"
55 },
56 {
57 "name": "Promortyus"
58 },
59 {
60 "name": "The Vat of Acid Episode"
61 },
62 {
63 "name": "Childrick of Mort"
64 }
65 ],
66 "info": {
67 "pages": 3
68 }
69 }
70 }
71}
Now lets try to get a little more complicated. How do we get the names of all the characters in a particular episode? Lets try to get the names of all the characters in the episode "Rixty Minutes". Lets first try with the REST API.
REST
We first need to get the information for the correct episode and pull down all the information there.
1BASE_URL = 'https://rickandmortyapi.com/api'
2
3CHARACTER_ENDPOINT = '/character'
4LOCATION_ENDPOINT = '/location'
5EPISODE_ENDPOINT = '/episode'
6
7NAME_FILTER = '/?name='
8episode_name = 'Rixty' #We are just going to filter based on the name, so we don't need the whole title
9
10
11response = requests.get(BASE_URL + EPISODE_ENDPOINT + NAME_FILTER + episode_name)
12json_object = response.json()
13json_formatted = json.dumps(json_object, indent=4)
14print(json_formatted)
1{
2 "info": {
3 "count": 1,
4 "pages": 1,
5 "next": null,
6 "prev": null
7 },
8 "results": [
9 {
10 "id": 8,
11 "name": "Rixty Minutes",
12 "air_date": "March 17, 2014",
13 "episode": "S01E08",
14 "characters": [
15 "https://rickandmortyapi.com/api/character/1",
16 "https://rickandmortyapi.com/api/character/2",
17 "https://rickandmortyapi.com/api/character/3",
18 "https://rickandmortyapi.com/api/character/4",
19 "https://rickandmortyapi.com/api/character/5",
20 "https://rickandmortyapi.com/api/character/20",
21 "https://rickandmortyapi.com/api/character/28",
22 "https://rickandmortyapi.com/api/character/29",
23 "https://rickandmortyapi.com/api/character/34",
24 "https://rickandmortyapi.com/api/character/37",
25 "https://rickandmortyapi.com/api/character/54",
26 "https://rickandmortyapi.com/api/character/88",
27 "https://rickandmortyapi.com/api/character/91",
28 "https://rickandmortyapi.com/api/character/129",
29 "https://rickandmortyapi.com/api/character/134",
30 "https://rickandmortyapi.com/api/character/136",
31 "https://rickandmortyapi.com/api/character/145",
32 "https://rickandmortyapi.com/api/character/153",
33 "https://rickandmortyapi.com/api/character/157",
34 "https://rickandmortyapi.com/api/character/176",
35 "https://rickandmortyapi.com/api/character/183",
36 "https://rickandmortyapi.com/api/character/184",
37 "https://rickandmortyapi.com/api/character/195",
38 "https://rickandmortyapi.com/api/character/207",
39 "https://rickandmortyapi.com/api/character/214",
40 "https://rickandmortyapi.com/api/character/222",
41 "https://rickandmortyapi.com/api/character/250",
42 "https://rickandmortyapi.com/api/character/266",
43 "https://rickandmortyapi.com/api/character/277",
44 "https://rickandmortyapi.com/api/character/279",
45 "https://rickandmortyapi.com/api/character/314",
46 "https://rickandmortyapi.com/api/character/315",
47 "https://rickandmortyapi.com/api/character/316",
48 "https://rickandmortyapi.com/api/character/317",
49 "https://rickandmortyapi.com/api/character/318",
50 "https://rickandmortyapi.com/api/character/351",
51 "https://rickandmortyapi.com/api/character/358",
52 "https://rickandmortyapi.com/api/character/367",
53 "https://rickandmortyapi.com/api/character/370",
54 "https://rickandmortyapi.com/api/character/373",
55 "https://rickandmortyapi.com/api/character/402",
56 "https://rickandmortyapi.com/api/character/403",
57 "https://rickandmortyapi.com/api/character/404",
58 "https://rickandmortyapi.com/api/character/405",
59 "https://rickandmortyapi.com/api/character/406",
60 "https://rickandmortyapi.com/api/character/407",
61 "https://rickandmortyapi.com/api/character/408",
62 "https://rickandmortyapi.com/api/character/409",
63 "https://rickandmortyapi.com/api/character/410",
64 "https://rickandmortyapi.com/api/character/411",
65 "https://rickandmortyapi.com/api/character/412",
66 "https://rickandmortyapi.com/api/character/413",
67 "https://rickandmortyapi.com/api/character/414",
68 "https://rickandmortyapi.com/api/character/415",
69 "https://rickandmortyapi.com/api/character/416",
70 "https://rickandmortyapi.com/api/character/417",
71 "https://rickandmortyapi.com/api/character/418"
72 ],
73 "url": "https://rickandmortyapi.com/api/episode/8",
74 "created": "2017-11-10T12:56:34.543Z"
75 }
76 ]
77}
So now we have a list of references to all the characters in the episode, but none of their actual names. To get those names we can hit each endpoint provided, or we can try to get more info in a single query by grabbing all the character id
numbers and sending them over together. But first we need to get all the character id
s.
1character_urls = json_object['results'][0]['characters']
2character_ids = []
3
4for character in character_urls:
5 id_num = character[42:]
6 character_ids.append(id_num)
Now we have all the id
s stored in a list, we just have to send them over to the right API endpoint and in the right format.
1BASE_URL = 'https://rickandmortyapi.com/api'
2
3CHARACTER_ENDPOINT = '/character'
4
5response = requests.get(BASE_URL + CHARACTER_ENDPOINT + '/' + ','.join(character_ids))
6json_object = response.json()
7json_formatted = json.dumps(json_object, indent=4)
8print(json_formatted)
1[
2 {
3 "id": 1,
4 "name": "Rick Sanchez",
5 "status": "Alive",
6 "species": "Human",
7 "type": "",
8 "gender": "Male",
9 "origin": {
10 "name": "Earth (C-137)",
11 "url": "https://rickandmortyapi.com/api/location/1"
12 },
13 "location": {
14 "name": "Earth (Replacement Dimension)",
15 "url": "https://rickandmortyapi.com/api/location/20"
16 },
17 "image": "https://rickandmortyapi.com/api/character/avatar/1.jpeg",
18 "episode": [
19 "https://rickandmortyapi.com/api/episode/1",
20 "https://rickandmortyapi.com/api/episode/2",
21 "https://rickandmortyapi.com/api/episode/3",
22 "https://rickandmortyapi.com/api/episode/4",
23 "https://rickandmortyapi.com/api/episode/5",
24 "https://rickandmortyapi.com/api/episode/6",
25 "https://rickandmortyapi.com/api/episode/7",
26 "https://rickandmortyapi.com/api/episode/8",
27 "https://rickandmortyapi.com/api/episode/9",
28 "https://rickandmortyapi.com/api/episode/10",
29 "https://rickandmortyapi.com/api/episode/11",
30 "https://rickandmortyapi.com/api/episode/12",
31 "https://rickandmortyapi.com/api/episode/13",
32 "https://rickandmortyapi.com/api/episode/14",
33 "https://rickandmortyapi.com/api/episode/15",
34 "https://rickandmortyapi.com/api/episode/16",
35 "https://rickandmortyapi.com/api/episode/17",
36 "https://rickandmortyapi.com/api/episode/18",
37 "https://rickandmortyapi.com/api/episode/19",
38 "https://rickandmortyapi.com/api/episode/20",
39 "https://rickandmortyapi.com/api/episode/21",
40 "https://rickandmortyapi.com/api/episode/22",
41 "https://rickandmortyapi.com/api/episode/23",
42 "https://rickandmortyapi.com/api/episode/24",
43 "https://rickandmortyapi.com/api/episode/25",
44 "https://rickandmortyapi.com/api/episode/26",
45 "https://rickandmortyapi.com/api/episode/27",
46 "https://rickandmortyapi.com/api/episode/28",
47 "https://rickandmortyapi.com/api/episode/29",
48 "https://rickandmortyapi.com/api/episode/30",
49 "https://rickandmortyapi.com/api/episode/31",
50 "https://rickandmortyapi.com/api/episode/32",
51 "https://rickandmortyapi.com/api/episode/33",
52 "https://rickandmortyapi.com/api/episode/34",
53 "https://rickandmortyapi.com/api/episode/35",
54 "https://rickandmortyapi.com/api/episode/36",
55 "https://rickandmortyapi.com/api/episode/37",
56 "https://rickandmortyapi.com/api/episode/38",
57 "https://rickandmortyapi.com/api/episode/39",
58 "https://rickandmortyapi.com/api/episode/40",
59 "https://rickandmortyapi.com/api/episode/41"
60 ],
61 "url": "https://rickandmortyapi.com/api/character/1",
62 "created": "2017-11-04T18:48:46.250Z"
63 },
64 {
65 "id": 2,
66 "name": "Morty Smith",
67 "status": "Alive",
68 "species": "Human",
69 "type": "",
70 "gender": "Male",
71 "origin": {
72 "name": "Earth (C-137)",
73 "url": "https://rickandmortyapi.com/api/location/1"
74 },
75 "location": {
76 "name": "Earth (Replacement Dimension)",
77 "url": "https://rickandmortyapi.com/api/location/20"
78 },
79 "image": "https://rickandmortyapi.com/api/character/avatar/2.jpeg",
80 "episode": [
81 "https://rickandmortyapi.com/api/episode/1",
82 "https://rickandmortyapi.com/api/episode/2",
83 "https://rickandmortyapi.com/api/episode/3",
84 "https://rickandmortyapi.com/api/episode/4",
85 "https://rickandmortyapi.com/api/episode/5",
86 "https://rickandmortyapi.com/api/episode/6",
87 "https://rickandmortyapi.com/api/episode/7",
88 "https://rickandmortyapi.com/api/episode/8",
89 "https://rickandmortyapi.com/api/episode/9",
90 "https://rickandmortyapi.com/api/episode/10",
91 "https://rickandmortyapi.com/api/episode/11",
92 "https://rickandmortyapi.com/api/episode/12",
93 "https://rickandmortyapi.com/api/episode/13",
94 "https://rickandmortyapi.com/api/episode/14",
95 "https://rickandmortyapi.com/api/episode/15",
96 "https://rickandmortyapi.com/api/episode/16",
97 "https://rickandmortyapi.com/api/episode/17",
98 "https://rickandmortyapi.com/api/episode/18",
99 "https://rickandmortyapi.com/api/episode/19",
100 "https://rickandmortyapi.com/api/episode/20",
101 "https://rickandmortyapi.com/api/episode/21",
102 "https://rickandmortyapi.com/api/episode/22",
103 "https://rickandmortyapi.com/api/episode/23",
104 "https://rickandmortyapi.com/api/episode/24",
105 "https://rickandmortyapi.com/api/episode/25",
106 "https://rickandmortyapi.com/api/episode/26",
107 "https://rickandmortyapi.com/api/episode/27",
108 "https://rickandmortyapi.com/api/episode/28",
109 "https://rickandmortyapi.com/api/episode/29",
110 "https://rickandmortyapi.com/api/episode/30",
111 "https://rickandmortyapi.com/api/episode/31",
112 "https://rickandmortyapi.com/api/episode/32",
113 "https://rickandmortyapi.com/api/episode/33",
114 "https://rickandmortyapi.com/api/episode/34",
115 "https://rickandmortyapi.com/api/episode/35",
116 "https://rickandmortyapi.com/api/episode/36",
117 "https://rickandmortyapi.com/api/episode/37",
118 "https://rickandmortyapi.com/api/episode/38",
119 "https://rickandmortyapi.com/api/episode/39",
120 "https://rickandmortyapi.com/api/episode/40",
121 "https://rickandmortyapi.com/api/episode/41"
122 ],
123 "url": "https://rickandmortyapi.com/api/character/2",
124 "created": "2017-11-04T18:50:21.651Z"
125 },
126 {
127 "id": 3,
128 "name": "Summer Smith",
129 "status": "Alive",
130 "species": "Human",
131 "type": "",
132 "gender": "Female",
133 "origin": {
134 "name": "Earth (Replacement Dimension)",
135 "url": "https://rickandmortyapi.com/api/location/20"
136 },
137 "location": {
138 "name": "Earth (Replacement Dimension)",
139 "url": "https://rickandmortyapi.com/api/location/20"
140 },
141 "image": "https://rickandmortyapi.com/api/character/avatar/3.jpeg",
142 "episode": [
143 "https://rickandmortyapi.com/api/episode/6",
144 "https://rickandmortyapi.com/api/episode/7",
145 "https://rickandmortyapi.com/api/episode/8",
146 "https://rickandmortyapi.com/api/episode/9",
147 "https://rickandmortyapi.com/api/episode/10",
148 "https://rickandmortyapi.com/api/episode/11",
149 "https://rickandmortyapi.com/api/episode/12",
150 "https://rickandmortyapi.com/api/episode/14",
151 "https://rickandmortyapi.com/api/episode/15",
152 "https://rickandmortyapi.com/api/episode/16",
153 "https://rickandmortyapi.com/api/episode/17",
154 "https://rickandmortyapi.com/api/episode/18",
155 "https://rickandmortyapi.com/api/episode/19",
156 "https://rickandmortyapi.com/api/episode/20",
157 "https://rickandmortyapi.com/api/episode/21",
158 "https://rickandmortyapi.com/api/episode/22",
159 "https://rickandmortyapi.com/api/episode/23",
160 "https://rickandmortyapi.com/api/episode/24",
161 "https://rickandmortyapi.com/api/episode/25",
162 "https://rickandmortyapi.com/api/episode/26",
163 "https://rickandmortyapi.com/api/episode/27",
164 "https://rickandmortyapi.com/api/episode/29",
165 "https://rickandmortyapi.com/api/episode/30",
166 "https://rickandmortyapi.com/api/episode/31",
167 "https://rickandmortyapi.com/api/episode/32",
168 "https://rickandmortyapi.com/api/episode/33",
169 "https://rickandmortyapi.com/api/episode/34",
170 "https://rickandmortyapi.com/api/episode/35",
171 "https://rickandmortyapi.com/api/episode/36",
172 "https://rickandmortyapi.com/api/episode/38",
173 "https://rickandmortyapi.com/api/episode/39",
174 "https://rickandmortyapi.com/api/episode/40",
175 "https://rickandmortyapi.com/api/episode/41"
176 ],
177 "url": "https://rickandmortyapi.com/api/character/3",
178 "created": "2017-11-04T19:09:56.428Z"
179 },
180 {
181 "id": 4,
182 "name": "Beth Smith",
183 "status": "Alive",
184 "species": "Human",
185 "type": "",
186 "gender": "Female",
187 "origin": {
188 "name": "Earth (Replacement Dimension)",
189 "url": "https://rickandmortyapi.com/api/location/20"
190 },
191 "location": {
192 "name": "Earth (Replacement Dimension)",
193 "url": "https://rickandmortyapi.com/api/location/20"
194 },
195 "image": "https://rickandmortyapi.com/api/character/avatar/4.jpeg",
196 "episode": [
197 "https://rickandmortyapi.com/api/episode/6",
198 "https://rickandmortyapi.com/api/episode/7",
199 "https://rickandmortyapi.com/api/episode/8",
200 "https://rickandmortyapi.com/api/episode/9",
201 "https://rickandmortyapi.com/api/episode/10",
202 "https://rickandmortyapi.com/api/episode/11",
203 "https://rickandmortyapi.com/api/episode/12",
204 "https://rickandmortyapi.com/api/episode/14",
205 "https://rickandmortyapi.com/api/episode/15",
206 "https://rickandmortyapi.com/api/episode/16",
207 "https://rickandmortyapi.com/api/episode/18",
208 "https://rickandmortyapi.com/api/episode/19",
209 "https://rickandmortyapi.com/api/episode/20",
210 "https://rickandmortyapi.com/api/episode/21",
211 "https://rickandmortyapi.com/api/episode/22",
212 "https://rickandmortyapi.com/api/episode/23",
213 "https://rickandmortyapi.com/api/episode/24",
214 "https://rickandmortyapi.com/api/episode/25",
215 "https://rickandmortyapi.com/api/episode/26",
216 "https://rickandmortyapi.com/api/episode/27",
217 "https://rickandmortyapi.com/api/episode/28",
218 "https://rickandmortyapi.com/api/episode/29",
219 "https://rickandmortyapi.com/api/episode/30",
220 "https://rickandmortyapi.com/api/episode/31",
221 "https://rickandmortyapi.com/api/episode/32",
222 "https://rickandmortyapi.com/api/episode/33",
223 "https://rickandmortyapi.com/api/episode/34",
224 "https://rickandmortyapi.com/api/episode/35",
225 "https://rickandmortyapi.com/api/episode/36",
226 "https://rickandmortyapi.com/api/episode/38",
227 "https://rickandmortyapi.com/api/episode/39",
228 "https://rickandmortyapi.com/api/episode/40",
229 "https://rickandmortyapi.com/api/episode/41"
230 ],
231 "url": "https://rickandmortyapi.com/api/character/4",
232 "created": "2017-11-04T19:22:43.665Z"
233 },
234 {
235 "id": 5,
236 "name": "Jerry Smith",
237 "status": "Alive",
238 "species": "Human",
239 "type": "",
240 "gender": "Male",
241 "origin": {
242 "name": "Earth (Replacement Dimension)",
243 "url": "https://rickandmortyapi.com/api/location/20"
244 },
245 "location": {
246 "name": "Earth (Replacement Dimension)",
247 "url": "https://rickandmortyapi.com/api/location/20"
248 },
249 "image": "https://rickandmortyapi.com/api/character/avatar/5.jpeg",
250 "episode": [
251 "https://rickandmortyapi.com/api/episode/6",
252 "https://rickandmortyapi.com/api/episode/7",
253 "https://rickandmortyapi.com/api/episode/8",
254 "https://rickandmortyapi.com/api/episode/9",
255 "https://rickandmortyapi.com/api/episode/10",
256 "https://rickandmortyapi.com/api/episode/11",
257 "https://rickandmortyapi.com/api/episode/12",
258 "https://rickandmortyapi.com/api/episode/13",
259 "https://rickandmortyapi.com/api/episode/14",
260 "https://rickandmortyapi.com/api/episode/15",
261 "https://rickandmortyapi.com/api/episode/16",
262 "https://rickandmortyapi.com/api/episode/18",
263 "https://rickandmortyapi.com/api/episode/19",
264 "https://rickandmortyapi.com/api/episode/20",
265 "https://rickandmortyapi.com/api/episode/21",
266 "https://rickandmortyapi.com/api/episode/22",
267 "https://rickandmortyapi.com/api/episode/23",
268 "https://rickandmortyapi.com/api/episode/26",
269 "https://rickandmortyapi.com/api/episode/29",
270 "https://rickandmortyapi.com/api/episode/30",
271 "https://rickandmortyapi.com/api/episode/31",
272 "https://rickandmortyapi.com/api/episode/32",
273 "https://rickandmortyapi.com/api/episode/33",
274 "https://rickandmortyapi.com/api/episode/35",
275 "https://rickandmortyapi.com/api/episode/36",
276 "https://rickandmortyapi.com/api/episode/38",
277 "https://rickandmortyapi.com/api/episode/39",
278 "https://rickandmortyapi.com/api/episode/40",
279 "https://rickandmortyapi.com/api/episode/41"
280 ],
281 "url": "https://rickandmortyapi.com/api/character/5",
282 "created": "2017-11-04T19:26:56.301Z"
283 },
284 {
285 "id": 20,
286 "name": "Ants in my Eyes Johnson",
287 "status": "unknown",
288 "species": "Human",
289 "type": "Human with ants in his eyes",
290 "gender": "Male",
291 "origin": {
292 "name": "unknown",
293 "url": ""
294 },
295 "location": {
296 "name": "Interdimensional Cable",
297 "url": "https://rickandmortyapi.com/api/location/6"
298 },
299 "image": "https://rickandmortyapi.com/api/character/avatar/20.jpeg",
300 "episode": [
301 "https://rickandmortyapi.com/api/episode/8"
302 ],
303 "url": "https://rickandmortyapi.com/api/character/20",
304 "created": "2017-11-04T22:34:53.659Z"
305 },
306 {
307 "id": 28,
308 "name": "Attila Starwar",
309 "status": "Alive",
310 "species": "Human",
311 "type": "",
312 "gender": "Male",
313 "origin": {
314 "name": "unknown",
315 "url": ""
316 },
317 "location": {
318 "name": "Interdimensional Cable",
319 "url": "https://rickandmortyapi.com/api/location/6"
320 },
321 "image": "https://rickandmortyapi.com/api/character/avatar/28.jpeg",
322 "episode": [
323 "https://rickandmortyapi.com/api/episode/8",
324 "https://rickandmortyapi.com/api/episode/13",
325 "https://rickandmortyapi.com/api/episode/17"
326 ],
327 "url": "https://rickandmortyapi.com/api/character/28",
328 "created": "2017-11-05T09:02:16.595Z"
329 },
330 {
331 "id": 29,
332 "name": "Baby Legs",
333 "status": "Alive",
334 "species": "Human",
335 "type": "Human with baby legs",
336 "gender": "Male",
337 "origin": {
338 "name": "unknown",
339 "url": ""
340 },
341 "location": {
342 "name": "Interdimensional Cable",
343 "url": "https://rickandmortyapi.com/api/location/6"
344 },
345 "image": "https://rickandmortyapi.com/api/character/avatar/29.jpeg",
346 "episode": [
347 "https://rickandmortyapi.com/api/episode/8"
348 ],
349 "url": "https://rickandmortyapi.com/api/character/29",
350 "created": "2017-11-05T09:06:19.644Z"
351 },
352 {
353 "id": 34,
354 "name": "Benjamin",
355 "status": "Alive",
356 "species": "Poopybutthole",
357 "type": "",
358 "gender": "Male",
359 "origin": {
360 "name": "unknown",
361 "url": ""
362 },
363 "location": {
364 "name": "Interdimensional Cable",
365 "url": "https://rickandmortyapi.com/api/location/6"
366 },
367 "image": "https://rickandmortyapi.com/api/character/avatar/34.jpeg",
368 "episode": [
369 "https://rickandmortyapi.com/api/episode/8",
370 "https://rickandmortyapi.com/api/episode/13",
371 "https://rickandmortyapi.com/api/episode/17"
372 ],
373 "url": "https://rickandmortyapi.com/api/character/34",
374 "created": "2017-11-05T09:24:04.748Z"
375 },
376 {
377 "id": 37,
378 "name": "Beth Sanchez",
379 "status": "Alive",
380 "species": "Human",
381 "type": "",
382 "gender": "Female",
383 "origin": {
384 "name": "Earth (C-500A)",
385 "url": "https://rickandmortyapi.com/api/location/23"
386 },
387 "location": {
388 "name": "Earth (C-500A)",
389 "url": "https://rickandmortyapi.com/api/location/23"
390 },
391 "image": "https://rickandmortyapi.com/api/character/avatar/37.jpeg",
392 "episode": [
393 "https://rickandmortyapi.com/api/episode/8"
394 ],
395 "url": "https://rickandmortyapi.com/api/character/37",
396 "created": "2017-11-05T09:38:22.960Z"
397 },
398 {
399 "id": 54,
400 "name": "Bobby Moynihan",
401 "status": "Alive",
402 "species": "Human",
403 "type": "",
404 "gender": "Male",
405 "origin": {
406 "name": "unknown",
407 "url": ""
408 },
409 "location": {
410 "name": "Interdimensional Cable",
411 "url": "https://rickandmortyapi.com/api/location/6"
412 },
413 "image": "https://rickandmortyapi.com/api/character/avatar/54.jpeg",
414 "episode": [
415 "https://rickandmortyapi.com/api/episode/8"
416 ],
417 "url": "https://rickandmortyapi.com/api/character/54",
418 "created": "2017-11-05T11:31:26.348Z"
419 },
420 {
421 "id": 88,
422 "name": "Cynthia",
423 "status": "Alive",
424 "species": "Human",
425 "type": "",
426 "gender": "Female",
427 "origin": {
428 "name": "Earth (Replacement Dimension)",
429 "url": "https://rickandmortyapi.com/api/location/20"
430 },
431 "location": {
432 "name": "Earth (Replacement Dimension)",
433 "url": "https://rickandmortyapi.com/api/location/20"
434 },
435 "image": "https://rickandmortyapi.com/api/character/avatar/88.jpeg",
436 "episode": [
437 "https://rickandmortyapi.com/api/episode/8",
438 "https://rickandmortyapi.com/api/episode/9",
439 "https://rickandmortyapi.com/api/episode/11",
440 "https://rickandmortyapi.com/api/episode/25"
441 ],
442 "url": "https://rickandmortyapi.com/api/character/88",
443 "created": "2017-11-30T21:16:35.633Z"
444 },
445 {
446 "id": 91,
447 "name": "David Letterman",
448 "status": "Alive",
449 "species": "Human",
450 "type": "",
451 "gender": "Male",
452 "origin": {
453 "name": "Earth (C-500A)",
454 "url": "https://rickandmortyapi.com/api/location/23"
455 },
456 "location": {
457 "name": "Earth (C-500A)",
458 "url": "https://rickandmortyapi.com/api/location/23"
459 },
460 "image": "https://rickandmortyapi.com/api/character/avatar/91.jpeg",
461 "episode": [
462 "https://rickandmortyapi.com/api/episode/8"
463 ],
464 "url": "https://rickandmortyapi.com/api/character/91",
465 "created": "2017-12-01T11:12:25.105Z"
466 },
467 {
468 "id": 129,
469 "name": "Fulgora",
470 "status": "Alive",
471 "species": "Human",
472 "type": "",
473 "gender": "Female",
474 "origin": {
475 "name": "unknown",
476 "url": ""
477 },
478 "location": {
479 "name": "Interdimensional Cable",
480 "url": "https://rickandmortyapi.com/api/location/6"
481 },
482 "image": "https://rickandmortyapi.com/api/character/avatar/129.jpeg",
483 "episode": [
484 "https://rickandmortyapi.com/api/episode/8",
485 "https://rickandmortyapi.com/api/episode/13",
486 "https://rickandmortyapi.com/api/episode/17"
487 ],
488 "url": "https://rickandmortyapi.com/api/character/129",
489 "created": "2017-12-26T19:30:02.242Z"
490 },
491 {
492 "id": 134,
493 "name": "Garmanarnar",
494 "status": "Alive",
495 "species": "Alien",
496 "type": "",
497 "gender": "Male",
498 "origin": {
499 "name": "unknown",
500 "url": ""
501 },
502 "location": {
503 "name": "Interdimensional Cable",
504 "url": "https://rickandmortyapi.com/api/location/6"
505 },
506 "image": "https://rickandmortyapi.com/api/character/avatar/134.jpeg",
507 "episode": [
508 "https://rickandmortyapi.com/api/episode/8"
509 ],
510 "url": "https://rickandmortyapi.com/api/character/134",
511 "created": "2017-12-26T20:36:54.577Z"
512 },
513 {
514 "id": 136,
515 "name": "Gazorpazorpfield",
516 "status": "Alive",
517 "species": "Alien",
518 "type": "Gazorpian",
519 "gender": "Male",
520 "origin": {
521 "name": "Gazorpazorp",
522 "url": "https://rickandmortyapi.com/api/location/40"
523 },
524 "location": {
525 "name": "Interdimensional Cable",
526 "url": "https://rickandmortyapi.com/api/location/6"
527 },
528 "image": "https://rickandmortyapi.com/api/character/avatar/136.jpeg",
529 "episode": [
530 "https://rickandmortyapi.com/api/episode/8",
531 "https://rickandmortyapi.com/api/episode/13"
532 ],
533 "url": "https://rickandmortyapi.com/api/character/136",
534 "created": "2017-12-27T17:59:59.058Z"
535 },
536 {
537 "id": 145,
538 "name": "Glenn",
539 "status": "Alive",
540 "species": "Human",
541 "type": "Eat shiter-Person",
542 "gender": "Male",
543 "origin": {
544 "name": "unknown",
545 "url": ""
546 },
547 "location": {
548 "name": "Interdimensional Cable",
549 "url": "https://rickandmortyapi.com/api/location/6"
550 },
551 "image": "https://rickandmortyapi.com/api/character/avatar/145.jpeg",
552 "episode": [
553 "https://rickandmortyapi.com/api/episode/8"
554 ],
555 "url": "https://rickandmortyapi.com/api/character/145",
556 "created": "2017-12-29T11:03:43.118Z"
557 },
558 {
559 "id": 153,
560 "name": "Hamster In Butt",
561 "status": "Alive",
562 "species": "Animal",
563 "type": "",
564 "gender": "unknown",
565 "origin": {
566 "name": "Hamster in Butt World",
567 "url": "https://rickandmortyapi.com/api/location/41"
568 },
569 "location": {
570 "name": "Hamster in Butt World",
571 "url": "https://rickandmortyapi.com/api/location/41"
572 },
573 "image": "https://rickandmortyapi.com/api/character/avatar/153.jpeg",
574 "episode": [
575 "https://rickandmortyapi.com/api/episode/8",
576 "https://rickandmortyapi.com/api/episode/19"
577 ],
578 "url": "https://rickandmortyapi.com/api/character/153",
579 "created": "2017-12-29T15:27:54.281Z"
580 },
581 {
582 "id": 157,
583 "name": "Hole in the Wall Where the Men Can See it All",
584 "status": "unknown",
585 "species": "unknown",
586 "type": "Hole",
587 "gender": "Genderless",
588 "origin": {
589 "name": "unknown",
590 "url": ""
591 },
592 "location": {
593 "name": "Interdimensional Cable",
594 "url": "https://rickandmortyapi.com/api/location/6"
595 },
596 "image": "https://rickandmortyapi.com/api/character/avatar/157.jpeg",
597 "episode": [
598 "https://rickandmortyapi.com/api/episode/8"
599 ],
600 "url": "https://rickandmortyapi.com/api/character/157",
601 "created": "2017-12-29T15:47:57.352Z"
602 },
603 {
604 "id": 176,
605 "name": "Celebrity Jerry",
606 "status": "Alive",
607 "species": "Human",
608 "type": "",
609 "gender": "Male",
610 "origin": {
611 "name": "Earth (C-500A)",
612 "url": "https://rickandmortyapi.com/api/location/23"
613 },
614 "location": {
615 "name": "Earth (C-500A)",
616 "url": "https://rickandmortyapi.com/api/location/23"
617 },
618 "image": "https://rickandmortyapi.com/api/character/avatar/176.jpeg",
619 "episode": [
620 "https://rickandmortyapi.com/api/episode/8"
621 ],
622 "url": "https://rickandmortyapi.com/api/character/176",
623 "created": "2017-12-29T18:25:11.930Z"
624 },
625 {
626 "id": 183,
627 "name": "Johnny Depp",
628 "status": "Alive",
629 "species": "Human",
630 "type": "",
631 "gender": "Male",
632 "origin": {
633 "name": "Earth (C-500A)",
634 "url": "https://rickandmortyapi.com/api/location/23"
635 },
636 "location": {
637 "name": "Earth (C-500A)",
638 "url": "https://rickandmortyapi.com/api/location/23"
639 },
640 "image": "https://rickandmortyapi.com/api/character/avatar/183.jpeg",
641 "episode": [
642 "https://rickandmortyapi.com/api/episode/8"
643 ],
644 "url": "https://rickandmortyapi.com/api/character/183",
645 "created": "2017-12-29T18:51:29.693Z"
646 },
647 {
648 "id": 184,
649 "name": "Jon",
650 "status": "Alive",
651 "species": "Alien",
652 "type": "Gazorpian",
653 "gender": "Male",
654 "origin": {
655 "name": "Gazorpazorp",
656 "url": "https://rickandmortyapi.com/api/location/40"
657 },
658 "location": {
659 "name": "Interdimensional Cable",
660 "url": "https://rickandmortyapi.com/api/location/6"
661 },
662 "image": "https://rickandmortyapi.com/api/character/avatar/184.jpeg",
663 "episode": [
664 "https://rickandmortyapi.com/api/episode/8"
665 ],
666 "url": "https://rickandmortyapi.com/api/character/184",
667 "created": "2017-12-29T18:54:04.572Z"
668 },
669 {
670 "id": 195,
671 "name": "Kristen Stewart",
672 "status": "Alive",
673 "species": "Human",
674 "type": "",
675 "gender": "Female",
676 "origin": {
677 "name": "Earth (C-500A)",
678 "url": "https://rickandmortyapi.com/api/location/23"
679 },
680 "location": {
681 "name": "Earth (C-500A)",
682 "url": "https://rickandmortyapi.com/api/location/23"
683 },
684 "image": "https://rickandmortyapi.com/api/character/avatar/195.jpeg",
685 "episode": [
686 "https://rickandmortyapi.com/api/episode/8"
687 ],
688 "url": "https://rickandmortyapi.com/api/character/195",
689 "created": "2017-12-30T12:19:16.042Z"
690 },
691 {
692 "id": 207,
693 "name": "Loggins",
694 "status": "Alive",
695 "species": "Alien",
696 "type": "Alligator-Person",
697 "gender": "Male",
698 "origin": {
699 "name": "unknown",
700 "url": ""
701 },
702 "location": {
703 "name": "Interdimensional Cable",
704 "url": "https://rickandmortyapi.com/api/location/6"
705 },
706 "image": "https://rickandmortyapi.com/api/character/avatar/207.jpeg",
707 "episode": [
708 "https://rickandmortyapi.com/api/episode/8",
709 "https://rickandmortyapi.com/api/episode/13",
710 "https://rickandmortyapi.com/api/episode/17"
711 ],
712 "url": "https://rickandmortyapi.com/api/character/207",
713 "created": "2017-12-30T13:54:28.627Z"
714 },
715 {
716 "id": 214,
717 "name": "Man Painted Silver Who Makes Robot Noises",
718 "status": "Alive",
719 "species": "Human",
720 "type": "",
721 "gender": "Male",
722 "origin": {
723 "name": "unknown",
724 "url": ""
725 },
726 "location": {
727 "name": "Interdimensional Cable",
728 "url": "https://rickandmortyapi.com/api/location/6"
729 },
730 "image": "https://rickandmortyapi.com/api/character/avatar/214.jpeg",
731 "episode": [
732 "https://rickandmortyapi.com/api/episode/8"
733 ],
734 "url": "https://rickandmortyapi.com/api/character/214",
735 "created": "2017-12-30T14:24:57.993Z"
736 },
737 {
738 "id": 222,
739 "name": "Michael Denny and the Denny Singers",
740 "status": "Alive",
741 "species": "Human",
742 "type": "",
743 "gender": "Male",
744 "origin": {
745 "name": "unknown",
746 "url": ""
747 },
748 "location": {
749 "name": "Interdimensional Cable",
750 "url": "https://rickandmortyapi.com/api/location/6"
751 },
752 "image": "https://rickandmortyapi.com/api/character/avatar/222.jpeg",
753 "episode": [
754 "https://rickandmortyapi.com/api/episode/8"
755 ],
756 "url": "https://rickandmortyapi.com/api/character/222",
757 "created": "2017-12-30T14:44:05.245Z"
758 },
759 {
760 "id": 250,
761 "name": "Mrs. Sullivan",
762 "status": "Dead",
763 "species": "Human",
764 "type": "Cat controlled dead lady",
765 "gender": "Female",
766 "origin": {
767 "name": "Earth (C-500A)",
768 "url": "https://rickandmortyapi.com/api/location/23"
769 },
770 "location": {
771 "name": "Interdimensional Cable",
772 "url": "https://rickandmortyapi.com/api/location/6"
773 },
774 "image": "https://rickandmortyapi.com/api/character/avatar/250.jpeg",
775 "episode": [
776 "https://rickandmortyapi.com/api/episode/8"
777 ],
778 "url": "https://rickandmortyapi.com/api/character/250",
779 "created": "2017-12-30T18:24:49.372Z"
780 },
781 {
782 "id": 266,
783 "name": "Piece of Toast",
784 "status": "Alive",
785 "species": "unknown",
786 "type": "Bread",
787 "gender": "Genderless",
788 "origin": {
789 "name": "unknown",
790 "url": ""
791 },
792 "location": {
793 "name": "Interdimensional Cable",
794 "url": "https://rickandmortyapi.com/api/location/6"
795 },
796 "image": "https://rickandmortyapi.com/api/character/avatar/266.jpeg",
797 "episode": [
798 "https://rickandmortyapi.com/api/episode/8",
799 "https://rickandmortyapi.com/api/episode/17"
800 ],
801 "url": "https://rickandmortyapi.com/api/character/266",
802 "created": "2017-12-31T13:48:58.850Z"
803 },
804 {
805 "id": 277,
806 "name": "Real Fake Doors Salesman",
807 "status": "Alive",
808 "species": "Human",
809 "type": "",
810 "gender": "Male",
811 "origin": {
812 "name": "unknown",
813 "url": ""
814 },
815 "location": {
816 "name": "Interdimensional Cable",
817 "url": "https://rickandmortyapi.com/api/location/6"
818 },
819 "image": "https://rickandmortyapi.com/api/character/avatar/277.jpeg",
820 "episode": [
821 "https://rickandmortyapi.com/api/episode/8"
822 ],
823 "url": "https://rickandmortyapi.com/api/character/277",
824 "created": "2017-12-31T14:18:30.570Z"
825 },
826 {
827 "id": 279,
828 "name": "Regular Legs",
829 "status": "Alive",
830 "species": "Human",
831 "type": "",
832 "gender": "Male",
833 "origin": {
834 "name": "unknown",
835 "url": ""
836 },
837 "location": {
838 "name": "Interdimensional Cable",
839 "url": "https://rickandmortyapi.com/api/location/6"
840 },
841 "image": "https://rickandmortyapi.com/api/character/avatar/279.jpeg",
842 "episode": [
843 "https://rickandmortyapi.com/api/episode/8"
844 ],
845 "url": "https://rickandmortyapi.com/api/character/279",
846 "created": "2017-12-31T14:20:31.936Z"
847 },
848 {
849 "id": 314,
850 "name": "Shmlamantha Shmlicelli",
851 "status": "Alive",
852 "species": "Human",
853 "type": "",
854 "gender": "Female",
855 "origin": {
856 "name": "unknown",
857 "url": ""
858 },
859 "location": {
860 "name": "Interdimensional Cable",
861 "url": "https://rickandmortyapi.com/api/location/6"
862 },
863 "image": "https://rickandmortyapi.com/api/character/avatar/314.jpeg",
864 "episode": [
865 "https://rickandmortyapi.com/api/episode/8"
866 ],
867 "url": "https://rickandmortyapi.com/api/character/314",
868 "created": "2018-01-05T14:53:23.739Z"
869 },
870 {
871 "id": 315,
872 "name": "Shmlangela Shmlobinson-Shmlower",
873 "status": "Alive",
874 "species": "Human",
875 "type": "",
876 "gender": "Female",
877 "origin": {
878 "name": "unknown",
879 "url": ""
880 },
881 "location": {
882 "name": "Interdimensional Cable",
883 "url": "https://rickandmortyapi.com/api/location/6"
884 },
885 "image": "https://rickandmortyapi.com/api/character/avatar/315.jpeg",
886 "episode": [
887 "https://rickandmortyapi.com/api/episode/8"
888 ],
889 "url": "https://rickandmortyapi.com/api/character/315",
890 "created": "2018-01-05T14:54:37.641Z"
891 },
892 {
893 "id": 316,
894 "name": "Shmlona Shmlobinson",
895 "status": "Alive",
896 "species": "Human",
897 "type": "",
898 "gender": "Female",
899 "origin": {
900 "name": "unknown",
901 "url": ""
902 },
903 "location": {
904 "name": "Interdimensional Cable",
905 "url": "https://rickandmortyapi.com/api/location/6"
906 },
907 "image": "https://rickandmortyapi.com/api/character/avatar/316.jpeg",
908 "episode": [
909 "https://rickandmortyapi.com/api/episode/8"
910 ],
911 "url": "https://rickandmortyapi.com/api/character/316",
912 "created": "2018-01-05T14:55:42.034Z"
913 },
914 {
915 "id": 317,
916 "name": "Shmlonathan Shmlower",
917 "status": "Alive",
918 "species": "Human",
919 "type": "",
920 "gender": "Male",
921 "origin": {
922 "name": "unknown",
923 "url": ""
924 },
925 "location": {
926 "name": "Interdimensional Cable",
927 "url": "https://rickandmortyapi.com/api/location/6"
928 },
929 "image": "https://rickandmortyapi.com/api/character/avatar/317.jpeg",
930 "episode": [
931 "https://rickandmortyapi.com/api/episode/8"
932 ],
933 "url": "https://rickandmortyapi.com/api/character/317",
934 "created": "2018-01-05T14:56:15.428Z"
935 },
936 {
937 "id": 318,
938 "name": "Shmlony Shmlicelli",
939 "status": "Alive",
940 "species": "Human",
941 "type": "",
942 "gender": "Male",
943 "origin": {
944 "name": "unknown",
945 "url": ""
946 },
947 "location": {
948 "name": "Interdimensional Cable",
949 "url": "https://rickandmortyapi.com/api/location/6"
950 },
951 "image": "https://rickandmortyapi.com/api/character/avatar/318.jpeg",
952 "episode": [
953 "https://rickandmortyapi.com/api/episode/8"
954 ],
955 "url": "https://rickandmortyapi.com/api/character/318",
956 "created": "2018-01-05T14:56:45.502Z"
957 },
958 {
959 "id": 351,
960 "name": "Three Unknown Things",
961 "status": "Alive",
962 "species": "Alien",
963 "type": "",
964 "gender": "unknown",
965 "origin": {
966 "name": "unknown",
967 "url": ""
968 },
969 "location": {
970 "name": "Interdimensional Cable",
971 "url": "https://rickandmortyapi.com/api/location/6"
972 },
973 "image": "https://rickandmortyapi.com/api/character/avatar/351.jpeg",
974 "episode": [
975 "https://rickandmortyapi.com/api/episode/8"
976 ],
977 "url": "https://rickandmortyapi.com/api/character/351",
978 "created": "2018-01-10T17:57:31.022Z"
979 },
980 {
981 "id": 358,
982 "name": "Tophat Jones",
983 "status": "Dead",
984 "species": "Mythological Creature",
985 "type": "Leprechaun",
986 "gender": "Male",
987 "origin": {
988 "name": "unknown",
989 "url": ""
990 },
991 "location": {
992 "name": "Interdimensional Cable",
993 "url": "https://rickandmortyapi.com/api/location/6"
994 },
995 "image": "https://rickandmortyapi.com/api/character/avatar/358.jpeg",
996 "episode": [
997 "https://rickandmortyapi.com/api/episode/8",
998 "https://rickandmortyapi.com/api/episode/18",
999 "https://rickandmortyapi.com/api/episode/21"
1000 ],
1001 "url": "https://rickandmortyapi.com/api/character/358",
1002 "created": "2018-01-10T18:09:34.482Z"
1003 },
1004 {
1005 "id": 367,
1006 "name": "Trunk Man",
1007 "status": "Alive",
1008 "species": "Humanoid",
1009 "type": "Trunk-Person",
1010 "gender": "Male",
1011 "origin": {
1012 "name": "Trunk World",
1013 "url": "https://rickandmortyapi.com/api/location/65"
1014 },
1015 "location": {
1016 "name": "Interdimensional Cable",
1017 "url": "https://rickandmortyapi.com/api/location/6"
1018 },
1019 "image": "https://rickandmortyapi.com/api/character/avatar/367.jpeg",
1020 "episode": [
1021 "https://rickandmortyapi.com/api/episode/8",
1022 "https://rickandmortyapi.com/api/episode/28"
1023 ],
1024 "url": "https://rickandmortyapi.com/api/character/367",
1025 "created": "2018-01-10T19:05:34.004Z"
1026 },
1027 {
1028 "id": 370,
1029 "name": "Two Guys with Handlebar Mustaches",
1030 "status": "Alive",
1031 "species": "Human",
1032 "type": "",
1033 "gender": "Male",
1034 "origin": {
1035 "name": "unknown",
1036 "url": ""
1037 },
1038 "location": {
1039 "name": "Interdimensional Cable",
1040 "url": "https://rickandmortyapi.com/api/location/6"
1041 },
1042 "image": "https://rickandmortyapi.com/api/character/avatar/370.jpeg",
1043 "episode": [
1044 "https://rickandmortyapi.com/api/episode/8"
1045 ],
1046 "url": "https://rickandmortyapi.com/api/character/370",
1047 "created": "2018-01-10T19:17:57.976Z"
1048 },
1049 {
1050 "id": 373,
1051 "name": "Unmuscular Michael",
1052 "status": "Alive",
1053 "species": "Human",
1054 "type": "",
1055 "gender": "Male",
1056 "origin": {
1057 "name": "unknown",
1058 "url": ""
1059 },
1060 "location": {
1061 "name": "Interdimensional Cable",
1062 "url": "https://rickandmortyapi.com/api/location/6"
1063 },
1064 "image": "https://rickandmortyapi.com/api/character/avatar/373.jpeg",
1065 "episode": [
1066 "https://rickandmortyapi.com/api/episode/8"
1067 ],
1068 "url": "https://rickandmortyapi.com/api/character/373",
1069 "created": "2018-01-10T19:22:04.075Z"
1070 },
1071 {
1072 "id": 402,
1073 "name": "Guy from The Bachelor",
1074 "status": "Alive",
1075 "species": "Human",
1076 "type": "",
1077 "gender": "Male",
1078 "origin": {
1079 "name": "Earth (Replacement Dimension)",
1080 "url": "https://rickandmortyapi.com/api/location/20"
1081 },
1082 "location": {
1083 "name": "Earth (Replacement Dimension)",
1084 "url": "https://rickandmortyapi.com/api/location/20"
1085 },
1086 "image": "https://rickandmortyapi.com/api/character/avatar/402.jpeg",
1087 "episode": [
1088 "https://rickandmortyapi.com/api/episode/8"
1089 ],
1090 "url": "https://rickandmortyapi.com/api/character/402",
1091 "created": "2018-04-15T17:45:38.589Z"
1092 },
1093 {
1094 "id": 403,
1095 "name": "Corn detective",
1096 "status": "Dead",
1097 "species": "Humanoid",
1098 "type": "Corn-person",
1099 "gender": "Male",
1100 "origin": {
1101 "name": "Interdimensional Cable",
1102 "url": "https://rickandmortyapi.com/api/location/6"
1103 },
1104 "location": {
1105 "name": "Interdimensional Cable",
1106 "url": "https://rickandmortyapi.com/api/location/6"
1107 },
1108 "image": "https://rickandmortyapi.com/api/character/avatar/403.jpeg",
1109 "episode": [
1110 "https://rickandmortyapi.com/api/episode/8"
1111 ],
1112 "url": "https://rickandmortyapi.com/api/character/403",
1113 "created": "2018-04-15T17:48:50.629Z"
1114 },
1115 {
1116 "id": 404,
1117 "name": "Michael Jackson",
1118 "status": "Alive",
1119 "species": "Humanoid",
1120 "type": "Phone-Person",
1121 "gender": "Male",
1122 "origin": {
1123 "name": "Earth (Phone Dimension)",
1124 "url": "https://rickandmortyapi.com/api/location/72"
1125 },
1126 "location": {
1127 "name": "Earth (Phone Dimension)",
1128 "url": "https://rickandmortyapi.com/api/location/72"
1129 },
1130 "image": "https://rickandmortyapi.com/api/character/avatar/404.jpeg",
1131 "episode": [
1132 "https://rickandmortyapi.com/api/episode/8"
1133 ],
1134 "url": "https://rickandmortyapi.com/api/character/404",
1135 "created": "2018-04-15T18:01:09.637Z"
1136 },
1137 {
1138 "id": 405,
1139 "name": "Trunkphobic suspenders guy",
1140 "status": "Alive",
1141 "species": "Human",
1142 "type": "",
1143 "gender": "Male",
1144 "origin": {
1145 "name": "unknown",
1146 "url": ""
1147 },
1148 "location": {
1149 "name": "Earth (Replacement Dimension)",
1150 "url": "https://rickandmortyapi.com/api/location/20"
1151 },
1152 "image": "https://rickandmortyapi.com/api/character/avatar/405.jpeg",
1153 "episode": [
1154 "https://rickandmortyapi.com/api/episode/2",
1155 "https://rickandmortyapi.com/api/episode/8",
1156 "https://rickandmortyapi.com/api/episode/10",
1157 "https://rickandmortyapi.com/api/episode/11"
1158 ],
1159 "url": "https://rickandmortyapi.com/api/character/405",
1160 "created": "2018-04-15T20:31:46.065Z"
1161 },
1162 {
1163 "id": 406,
1164 "name": "Spiderweb teddy bear",
1165 "status": "Alive",
1166 "species": "Animal",
1167 "type": "Teddy Bear",
1168 "gender": "unknown",
1169 "origin": {
1170 "name": "Interdimensional Cable",
1171 "url": "https://rickandmortyapi.com/api/location/6"
1172 },
1173 "location": {
1174 "name": "Interdimensional Cable",
1175 "url": "https://rickandmortyapi.com/api/location/6"
1176 },
1177 "image": "https://rickandmortyapi.com/api/character/avatar/406.jpeg",
1178 "episode": [
1179 "https://rickandmortyapi.com/api/episode/8"
1180 ],
1181 "url": "https://rickandmortyapi.com/api/character/406",
1182 "created": "2018-04-15T20:45:04.863Z"
1183 },
1184 {
1185 "id": 407,
1186 "name": "Regular Tyrion Lannister",
1187 "status": "Alive",
1188 "species": "Human",
1189 "type": "",
1190 "gender": "Male",
1191 "origin": {
1192 "name": "Interdimensional Cable",
1193 "url": "https://rickandmortyapi.com/api/location/6"
1194 },
1195 "location": {
1196 "name": "Interdimensional Cable",
1197 "url": "https://rickandmortyapi.com/api/location/6"
1198 },
1199 "image": "https://rickandmortyapi.com/api/character/avatar/407.jpeg",
1200 "episode": [
1201 "https://rickandmortyapi.com/api/episode/8"
1202 ],
1203 "url": "https://rickandmortyapi.com/api/character/407",
1204 "created": "2018-04-15T20:50:10.475Z"
1205 },
1206 {
1207 "id": 408,
1208 "name": "Quick Mystery Presenter",
1209 "status": "Alive",
1210 "species": "Human",
1211 "type": "",
1212 "gender": "Male",
1213 "origin": {
1214 "name": "Interdimensional Cable",
1215 "url": "https://rickandmortyapi.com/api/location/6"
1216 },
1217 "location": {
1218 "name": "Interdimensional Cable",
1219 "url": "https://rickandmortyapi.com/api/location/6"
1220 },
1221 "image": "https://rickandmortyapi.com/api/character/avatar/408.jpeg",
1222 "episode": [
1223 "https://rickandmortyapi.com/api/episode/8"
1224 ],
1225 "url": "https://rickandmortyapi.com/api/character/408",
1226 "created": "2018-04-15T20:51:47.778Z"
1227 },
1228 {
1229 "id": 409,
1230 "name": "Mr. Sneezy",
1231 "status": "Alive",
1232 "species": "Human",
1233 "type": "Little Human",
1234 "gender": "Male",
1235 "origin": {
1236 "name": "Interdimensional Cable",
1237 "url": "https://rickandmortyapi.com/api/location/6"
1238 },
1239 "location": {
1240 "name": "Interdimensional Cable",
1241 "url": "https://rickandmortyapi.com/api/location/6"
1242 },
1243 "image": "https://rickandmortyapi.com/api/character/avatar/409.jpeg",
1244 "episode": [
1245 "https://rickandmortyapi.com/api/episode/8"
1246 ],
1247 "url": "https://rickandmortyapi.com/api/character/409",
1248 "created": "2018-04-15T21:04:09.405Z"
1249 },
1250 {
1251 "id": 410,
1252 "name": "Two Brothers",
1253 "status": "Alive",
1254 "species": "Human",
1255 "type": "",
1256 "gender": "Male",
1257 "origin": {
1258 "name": "Interdimensional Cable",
1259 "url": "https://rickandmortyapi.com/api/location/6"
1260 },
1261 "location": {
1262 "name": "Interdimensional Cable",
1263 "url": "https://rickandmortyapi.com/api/location/6"
1264 },
1265 "image": "https://rickandmortyapi.com/api/character/avatar/410.jpeg",
1266 "episode": [
1267 "https://rickandmortyapi.com/api/episode/8"
1268 ],
1269 "url": "https://rickandmortyapi.com/api/character/410",
1270 "created": "2018-04-15T21:06:18.686Z"
1271 },
1272 {
1273 "id": 411,
1274 "name": "Alien Mexican Armada",
1275 "status": "unknown",
1276 "species": "Alien",
1277 "type": "Mexican",
1278 "gender": "Male",
1279 "origin": {
1280 "name": "Interdimensional Cable",
1281 "url": "https://rickandmortyapi.com/api/location/6"
1282 },
1283 "location": {
1284 "name": "Interdimensional Cable",
1285 "url": "https://rickandmortyapi.com/api/location/6"
1286 },
1287 "image": "https://rickandmortyapi.com/api/character/avatar/411.jpeg",
1288 "episode": [
1289 "https://rickandmortyapi.com/api/episode/8"
1290 ],
1291 "url": "https://rickandmortyapi.com/api/character/411",
1292 "created": "2018-04-15T21:10:27.986Z"
1293 },
1294 {
1295 "id": 412,
1296 "name": "Giant Cat Monster",
1297 "status": "unknown",
1298 "species": "Animal",
1299 "type": "Giant Cat Monster",
1300 "gender": "unknown",
1301 "origin": {
1302 "name": "Interdimensional Cable",
1303 "url": "https://rickandmortyapi.com/api/location/6"
1304 },
1305 "location": {
1306 "name": "Interdimensional Cable",
1307 "url": "https://rickandmortyapi.com/api/location/6"
1308 },
1309 "image": "https://rickandmortyapi.com/api/character/avatar/412.jpeg",
1310 "episode": [
1311 "https://rickandmortyapi.com/api/episode/8"
1312 ],
1313 "url": "https://rickandmortyapi.com/api/character/412",
1314 "created": "2018-04-15T21:14:09.223Z"
1315 },
1316 {
1317 "id": 413,
1318 "name": "Old Women",
1319 "status": "unknown",
1320 "species": "Human",
1321 "type": "Old Amazons",
1322 "gender": "Female",
1323 "origin": {
1324 "name": "Interdimensional Cable",
1325 "url": "https://rickandmortyapi.com/api/location/6"
1326 },
1327 "location": {
1328 "name": "Interdimensional Cable",
1329 "url": "https://rickandmortyapi.com/api/location/6"
1330 },
1331 "image": "https://rickandmortyapi.com/api/character/avatar/413.jpeg",
1332 "episode": [
1333 "https://rickandmortyapi.com/api/episode/8"
1334 ],
1335 "url": "https://rickandmortyapi.com/api/character/413",
1336 "created": "2018-04-15T21:21:32.623Z"
1337 },
1338 {
1339 "id": 414,
1340 "name": "Trunkphobic guy",
1341 "status": "Alive",
1342 "species": "Human",
1343 "type": "",
1344 "gender": "Male",
1345 "origin": {
1346 "name": "Interdimensional Cable",
1347 "url": "https://rickandmortyapi.com/api/location/6"
1348 },
1349 "location": {
1350 "name": "Interdimensional Cable",
1351 "url": "https://rickandmortyapi.com/api/location/6"
1352 },
1353 "image": "https://rickandmortyapi.com/api/character/avatar/414.jpeg",
1354 "episode": [
1355 "https://rickandmortyapi.com/api/episode/8"
1356 ],
1357 "url": "https://rickandmortyapi.com/api/character/414",
1358 "created": "2018-04-15T21:33:59.719Z"
1359 },
1360 {
1361 "id": 415,
1362 "name": "Pro trunk people marriage guy",
1363 "status": "Alive",
1364 "species": "Human",
1365 "type": "",
1366 "gender": "Male",
1367 "origin": {
1368 "name": "Interdimensional Cable",
1369 "url": "https://rickandmortyapi.com/api/location/6"
1370 },
1371 "location": {
1372 "name": "Interdimensional Cable",
1373 "url": "https://rickandmortyapi.com/api/location/6"
1374 },
1375 "image": "https://rickandmortyapi.com/api/character/avatar/415.jpeg",
1376 "episode": [
1377 "https://rickandmortyapi.com/api/episode/8"
1378 ],
1379 "url": "https://rickandmortyapi.com/api/character/415",
1380 "created": "2018-04-15T21:34:21.911Z"
1381 },
1382 {
1383 "id": 416,
1384 "name": "Muscular Mannie",
1385 "status": "Alive",
1386 "species": "Human",
1387 "type": "Mannie",
1388 "gender": "Male",
1389 "origin": {
1390 "name": "Interdimensional Cable",
1391 "url": "https://rickandmortyapi.com/api/location/6"
1392 },
1393 "location": {
1394 "name": "Interdimensional Cable",
1395 "url": "https://rickandmortyapi.com/api/location/6"
1396 },
1397 "image": "https://rickandmortyapi.com/api/character/avatar/416.jpeg",
1398 "episode": [
1399 "https://rickandmortyapi.com/api/episode/8"
1400 ],
1401 "url": "https://rickandmortyapi.com/api/character/416",
1402 "created": "2018-04-15T21:39:22.608Z"
1403 },
1404 {
1405 "id": 417,
1406 "name": "Baby Legs Chief",
1407 "status": "Alive",
1408 "species": "Human",
1409 "type": "",
1410 "gender": "Male",
1411 "origin": {
1412 "name": "Interdimensional Cable",
1413 "url": "https://rickandmortyapi.com/api/location/6"
1414 },
1415 "location": {
1416 "name": "Interdimensional Cable",
1417 "url": "https://rickandmortyapi.com/api/location/6"
1418 },
1419 "image": "https://rickandmortyapi.com/api/character/avatar/417.jpeg",
1420 "episode": [
1421 "https://rickandmortyapi.com/api/episode/8"
1422 ],
1423 "url": "https://rickandmortyapi.com/api/character/417",
1424 "created": "2018-04-15T21:40:39.871Z"
1425 },
1426 {
1427 "id": 418,
1428 "name": "Mrs. Sullivan's Boyfriend",
1429 "status": "Alive",
1430 "species": "Human",
1431 "type": "Necrophiliac",
1432 "gender": "Male",
1433 "origin": {
1434 "name": "Interdimensional Cable",
1435 "url": "https://rickandmortyapi.com/api/location/6"
1436 },
1437 "location": {
1438 "name": "Interdimensional Cable",
1439 "url": "https://rickandmortyapi.com/api/location/6"
1440 },
1441 "image": "https://rickandmortyapi.com/api/character/avatar/418.jpeg",
1442 "episode": [
1443 "https://rickandmortyapi.com/api/episode/8"
1444 ],
1445 "url": "https://rickandmortyapi.com/api/character/418",
1446 "created": "2018-04-15T21:43:43.867Z"
1447 }
1448]
So now we have all the characters in a list of objects, so now we just need to get all of their names.
1for character in json_object:
2 print(character['name'])
1Rick Sanchez
2Morty Smith
3Summer Smith
4Beth Smith
5Jerry Smith
6Ants in my Eyes Johnson
7Attila Starwar
8Baby Legs
9Benjamin
10Beth Sanchez
11Bobby Moynihan
12Cynthia
13David Letterman
14Fulgora
15Garmanarnar
16Gazorpazorpfield
17Glenn
18Hamster In Butt
19Hole in the Wall Where the Men Can See it All
20Celebrity Jerry
21Johnny Depp
22Jon
23Kristen Stewart
24Loggins
25Man Painted Silver Who Makes Robot Noises
26Michael Denny and the Denny Singers
27Mrs. Sullivan
28Piece of Toast
29Real Fake Doors Salesman
30Regular Legs
31Shmlamantha Shmlicelli
32Shmlangela Shmlobinson-Shmlower
33Shmlona Shmlobinson
34Shmlonathan Shmlower
35Shmlony Shmlicelli
36Three Unknown Things
37Tophat Jones
38Trunk Man
39Two Guys with Handlebar Mustaches
40Unmuscular Michael
41Guy from The Bachelor
42Corn detective
43Michael Jackson
44Trunkphobic suspenders guy
45Spiderweb teddy bear
46Regular Tyrion Lannister
47Quick Mystery Presenter
48Mr. Sneezy
49Two Brothers
50Alien Mexican Armada
51Giant Cat Monster
52Old Women
53Trunkphobic guy
54Pro trunk people marriage guy
55Muscular Mannie
56Baby Legs Chief
57Mrs. Sullivan's Boyfriend
58
It took some work, but we were able to get all the character's names in the episode "Rixty Minutes". Now lets see how to do the same thing in GraphQL.
GraphQL
First we need to graph the specific episode, so we are going to change our query a little bit.
1BASE_URL = 'https://rickandmortyapi.com/graphql'
2
3query = """ {
4 episodes(filter: { name: "Rixty Minutes" }) {
5 results {
6 name
7 id
8 }
9 }
10}
11"""
12
13response = requests.post(BASE_URL, json={'query': query})
14json_object = response.json()
15json_formatted = json.dumps(json_object, indent=4)
16print(json_formatted)
1{
2 "data": {
3 "episodes": {
4 "results": [
5 {
6 "name": "Rixty Minutes",
7 "id": "8"
8 }
9 ]
10 }
11 }
12}
So now we have the right episode, and the id. No we need to get all the characters names from that episode. But the thing is, we are approaching this problem like we are using a REST interface. We can actually skip a bunch of the steps that we had to go through with the REST process and just ask for the information that we want. So all we need to do is tell the GraphQL API that we also want all the characters in that episode, and then also their names. And to do that only takes two more lines in our query, and nothing more. And while we are at it, we don't actually care about the id
of the episode, so we can get rid of that.
1BASE_URL = 'https://rickandmortyapi.com/graphql'
2
3query = """ {
4 episodes(filter: { name: "Rixty Minutes" }) {
5 results {
6 name
7 characters {
8 name
9 }
10 }
11 }
12}
13"""
14
15response = requests.post(BASE_URL, json={'query': query})
16json_object = response.json()
17json_formatted = json.dumps(json_object, indent=4)
18print(json_formatted)
1{
2 "data": {
3 "episodes": {
4 "results": [
5 {
6 "name": "Rixty Minutes",
7 "characters": [
8 {
9 "name": "Rick Sanchez"
10 },
11 {
12 "name": "Morty Smith"
13 },
14 {
15 "name": "Summer Smith"
16 },
17 {
18 "name": "Beth Smith"
19 },
20 {
21 "name": "Jerry Smith"
22 },
23 {
24 "name": "Ants in my Eyes Johnson"
25 },
26 {
27 "name": "Attila Starwar"
28 },
29 {
30 "name": "Baby Legs"
31 },
32 {
33 "name": "Benjamin"
34 },
35 {
36 "name": "Beth Sanchez"
37 },
38 {
39 "name": "Bobby Moynihan"
40 },
41 {
42 "name": "Cynthia"
43 },
44 {
45 "name": "David Letterman"
46 },
47 {
48 "name": "Fulgora"
49 },
50 {
51 "name": "Garmanarnar"
52 },
53 {
54 "name": "Gazorpazorpfield"
55 },
56 {
57 "name": "Glenn"
58 },
59 {
60 "name": "Hamster In Butt"
61 },
62 {
63 "name": "Hole in the Wall Where the Men Can See it All"
64 },
65 {
66 "name": "Celebrity Jerry"
67 },
68 {
69 "name": "Johnny Depp"
70 },
71 {
72 "name": "Jon"
73 },
74 {
75 "name": "Kristen Stewart"
76 },
77 {
78 "name": "Loggins"
79 },
80 {
81 "name": "Man Painted Silver Who Makes Robot Noises"
82 },
83 {
84 "name": "Michael Denny and the Denny Singers"
85 },
86 {
87 "name": "Mrs. Sullivan"
88 },
89 {
90 "name": "Piece of Toast"
91 },
92 {
93 "name": "Real Fake Doors Salesman"
94 },
95 {
96 "name": "Regular Legs"
97 },
98 {
99 "name": "Shmlamantha Shmlicelli"
100 },
101 {
102 "name": "Shmlangela Shmlobinson-Shmlower"
103 },
104 {
105 "name": "Shmlona Shmlobinson"
106 },
107 {
108 "name": "Shmlonathan Shmlower"
109 },
110 {
111 "name": "Shmlony Shmlicelli"
112 },
113 {
114 "name": "Three Unknown Things"
115 },
116 {
117 "name": "Tophat Jones"
118 },
119 {
120 "name": "Trunk Man"
121 },
122 {
123 "name": "Two Guys with Handlebar Mustaches"
124 },
125 {
126 "name": "Unmuscular Michael"
127 },
128 {
129 "name": "Guy from The Bachelor"
130 },
131 {
132 "name": "Corn detective"
133 },
134 {
135 "name": "Michael Jackson"
136 },
137 {
138 "name": "Trunkphobic suspenders guy"
139 },
140 {
141 "name": "Spiderweb teddy bear"
142 },
143 {
144 "name": "Regular Tyrion Lannister"
145 },
146 {
147 "name": "Quick Mystery Presenter"
148 },
149 {
150 "name": "Mr. Sneezy"
151 },
152 {
153 "name": "Two Brothers"
154 },
155 {
156 "name": "Alien Mexican Armada"
157 },
158 {
159 "name": "Giant Cat Monster"
160 },
161 {
162 "name": "Old Women"
163 },
164 {
165 "name": "Trunkphobic guy"
166 },
167 {
168 "name": "Pro trunk people marriage guy"
169 },
170 {
171 "name": "Muscular Mannie"
172 },
173 {
174 "name": "Baby Legs Chief"
175 },
176 {
177 "name": "Mrs. Sullivan's Boyfriend"
178 }
179 ]
180 }
181 ]
182 }
183 }
184}
Now we have all the information that we want, and nothing else. We just have to dig through the JSON to get it.
1episode_characters = json_object['data']['episodes']['results'][0]['characters']
2
3for character in episode_characters:
4 print(character['name'])
1Rick Sanchez
2Morty Smith
3Summer Smith
4Beth Smith
5Jerry Smith
6Ants in my Eyes Johnson
7Attila Starwar
8Baby Legs
9Benjamin
10Beth Sanchez
11Bobby Moynihan
12Cynthia
13David Letterman
14Fulgora
15Garmanarnar
16Gazorpazorpfield
17Glenn
18Hamster In Butt
19Hole in the Wall Where the Men Can See it All
20Celebrity Jerry
21Johnny Depp
22Jon
23Kristen Stewart
24Loggins
25Man Painted Silver Who Makes Robot Noises
26Michael Denny and the Denny Singers
27Mrs. Sullivan
28Piece of Toast
29Real Fake Doors Salesman
30Regular Legs
31Shmlamantha Shmlicelli
32Shmlangela Shmlobinson-Shmlower
33Shmlona Shmlobinson
34Shmlonathan Shmlower
35Shmlony Shmlicelli
36Three Unknown Things
37Tophat Jones
38Trunk Man
39Two Guys with Handlebar Mustaches
40Unmuscular Michael
41Guy from The Bachelor
42Corn detective
43Michael Jackson
44Trunkphobic suspenders guy
45Spiderweb teddy bear
46Regular Tyrion Lannister
47Quick Mystery Presenter
48Mr. Sneezy
49Two Brothers
50Alien Mexican Armada
51Giant Cat Monster
52Old Women
53Trunkphobic guy
54Pro trunk people marriage guy
55Muscular Mannie
56Baby Legs Chief
57Mrs. Sullivan's Boyfriend
Though we were able to get all the information that we needed using both methods, I personally find the GraphQL way of doing things a whole lot easier. It is also a lot easier to add in more information or adjust it when you need to. And the nice playground makes development so much easier.
Hopefully this quick example implementation of both a REST interface and a GraphQL interface helped to demonstrate the differences between the two. As well as teach you how to use either to get the information that you need.